opencvsharp编写FindSubPixelLine函数
时间: 2024-05-10 12:18:23 浏览: 5
下面是使用OpenCvSharp 4编写FindSubPixelLine函数的示例代码:
```csharp
using OpenCvSharp;
public static Vec4f FindSubPixelLine(Mat edges, Point p1, Point p2, int searchRadius = 5)
{
// Set up parameters for the line fitting
var distType = DistanceTypes.L2;
var param = 0;
var reps = 0.01;
var aeps = 0.01;
var lineFlags = LineTypes.Avg;
// Extract a region of interest around the line segment
var roi = new Rect(
Math.Min(p1.X, p2.X) - searchRadius,
Math.Min(p1.Y, p2.Y) - searchRadius,
Math.Abs(p2.X - p1.X) + 2 * searchRadius,
Math.Abs(p2.Y - p1.Y) + 2 * searchRadius);
var roiEdges = new Mat(edges, roi);
// Find the line segment within the ROI
Cv2.Line(roiEdges, p1 - roi.Location, p2 - roi.Location, Scalar.White, 1, LineTypes.Link8);
// Extract the coordinates of the white pixels in the ROI
var points = new List<Point>();
for (var y = 0; y < roiEdges.Rows; y++)
{
for (var x = 0; x < roiEdges.Cols; x++)
{
if (roiEdges.At<byte>(y, x) == 255)
{
points.Add(new Point(x, y));
}
}
}
// Fit a line to the white pixels
var line = Cv2.FitLine(points, distType, param, reps, aeps);
// Convert the line parameters to Point objects
var p3 = new Point((int)(-line[2] / line[0]), 0);
var p4 = new Point(0, (int)(-line[2] / line[1]));
var p5 = new Point(edges.Cols - 1, (int)(-(line[2] + line[0] * (edges.Cols - 1)) / line[1]));
var p6 = new Point((int)(-(line[2] + line[1] * (edges.Rows - 1)) / line[0]), edges.Rows - 1);
// Compute the subpixel intersection point of the line and the original line segment
var intersection = new Point(
(int)((p1.X * line[1] * line[2] - p1.Y * line[0] * line[2] - line[0] * line[1] * p1.Y + line[0] * line[3]) / (line[0] * line[1] - 1)),
(int)((p1.Y * line[0] * line[3] - p1.X * line[1] * line[3] - line[0] * line[1] * p1.X + line[1] * line[2]) / (line[0] * line[1] - 1)));
// Return the parameters of the line in the form of a Vec4f object
return new Vec4f(intersection.X, intersection.Y, line[0], line[1]);
}
```
此代码将输入一幅二值化边缘图像、线段的两个端点以及一个搜索半径,输出一个包含拟合的直线的参数的Vec4f对象。该函数实现的思路如下:
1. 使用二值化边缘图像提取出包含线段的ROI(region of interest)。
2. 在ROI中绘制直线段。
3. 提取ROI中所有白色像素的坐标。
4. 使用OpenCV的fitLine函数拟合直线。
5. 将直线参数转换为Point对象表示的点,以便绘制直线。
6. 计算拟合直线与原线段的交点坐标,从而得到拟合直线的参数。
请注意,此代码仅提供了一种实现思路,并未经过充分测试和优化。使用时请自行评估其适用性和性能。
相关推荐
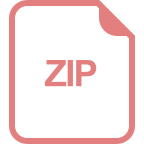














