react-router-dome v6如何做路由拦截
时间: 2023-08-30 17:06:30 浏览: 134
在 `react-router-dom` v6 中,路由拦截的方式与 v5 有所不同。下面是一个简单的示例:
```javascript
import React, { useState } from 'react';
import { BrowserRouter as Router, Routes, Route, Link, Navigate } from 'react-router-dom';
const isAuthenticated = true;
const PrivateRoute = ({ path, element }) => {
return isAuthenticated ? (
element
) : (
<Navigate to='/login' replace />
);
};
const LoginPage = () => (
<div>
<h1>Login Page</h1>
</div>
);
const HomePage = () => (
<div>
<h1>Home Page</h1>
</div>
);
const App = () => {
return (
<Router>
<div>
<ul>
<li><Link to='/'>Home</Link></li>
<li><Link to='/login'>Login</Link></li>
</ul>
<hr />
<Routes>
<PrivateRoute path='/' element={<HomePage />} />
<Route path='/login' element={<LoginPage />} />
</Routes>
</div>
</Router>
);
};
export default App;
```
在上面的代码中,我们定义了一个 `PrivateRoute` 组件来实现路由拦截。如果用户已经认证,则展示 `element` 组件,否则重定向到登录页面。
另外,我们还定义了两个页面组件:`HomePage` 和 `LoginPage`。在 `Routes` 组件中,我们使用 `PrivateRoute` 组件来保护 `HomePage` 组件,这样只有已经认证的用户才能访问该页面。
如果你想在路由跳转之前进行相关的操作,可以使用 `onBeforeChange` 函数。下面是一个简单的示例:
```javascript
import React, { useState } from 'react';
import { BrowserRouter as Router, Routes, Route, Link, Prompt } from 'react-router-dom';
const App = () => {
const [isBlocking, setIsBlocking] = useState(false);
const handleChange = (event) => {
setIsBlocking(event.target.value.length > 0);
};
const handleBeforeChange = (nextLocation) => {
if (isBlocking) {
return window.confirm(`Are you sure you want to go to ${nextLocation.pathname}?`);
}
return true;
};
return (
<Router>
<div>
<ul>
<li><Link to='/'>Home</Link></li>
<li><Link to='/about'>About</Link></li>
</ul>
<hr />
<Prompt
when={isBlocking}
message={location => `Are you sure you want to go to ${location.pathname}?`}
/>
<Routes onBeforeChange={handleBeforeChange}>
<Route path='/' element={<HomePage />} />
<Route path='/about' element={<AboutPage />} />
</Routes>
</div>
</Router>
);
};
export default App;
```
在上面的代码中,我们使用 `onBeforeChange` 函数来拦截路由,在用户输入内容时,如果尚未保存,就弹出提示框。如果用户选择离开当前页面,则路由会跳转到目标页面。
阅读全文
相关推荐
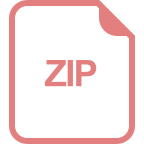
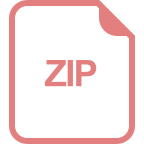
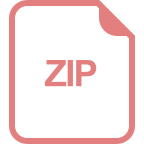
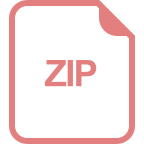
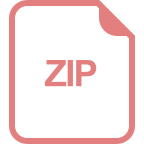
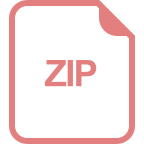
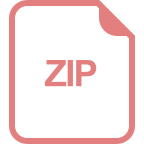
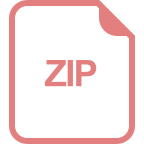
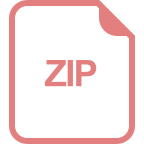
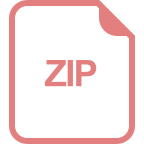
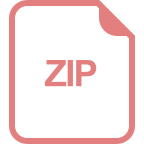
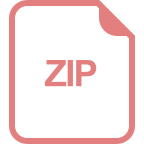
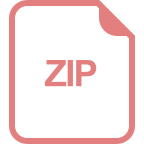
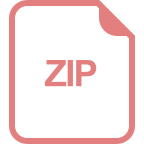
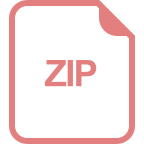
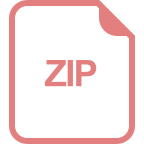
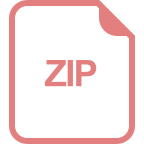
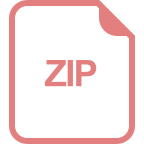
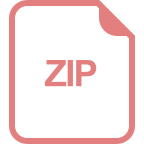