代码实现用nbiot和stm32发送数据1234和接受数据1234
时间: 2024-05-12 17:15:40 浏览: 105
这里给出一个简单的示例代码,使用NB-IoT模块和STM32微控制器发送和接收数据。
```c
#include "stm32f10x.h"
#include "stdio.h"
#define AT_CMD_DELAY_MS 500
void USART1_Init(void);
void USART1_SendChar(char ch);
void USART1_SendString(char* str);
void delay_ms(uint32_t ms);
void NB_IoT_Init(void);
void NB_IoT_SendData(char* data);
void NB_IoT_ReceiveData(void);
int main(void)
{
char data_to_send[] = "1234";
USART1_Init();
NB_IoT_Init();
while(1)
{
// Send data
NB_IoT_SendData(data_to_send);
delay_ms(AT_CMD_DELAY_MS);
// Receive data
NB_IoT_ReceiveData();
delay_ms(AT_CMD_DELAY_MS);
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// Enable GPIOA clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// Enable USART1 clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// Configure USART1 Tx (PA9) as alternate function push-pull
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1 Rx (PA10) as input floating
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1 parameters
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char ch)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(char* str)
{
while(*str)
{
USART1_SendChar(*str);
str++;
}
}
void delay_ms(uint32_t ms)
{
uint32_t i;
for(i=0; i<ms*1000; i++);
}
void NB_IoT_Init(void)
{
// Configure NB-IoT module
// ...
}
void NB_IoT_SendData(char* data)
{
USART1_SendString("AT+NSOST=0.0.0.0,5684,");
USART1_SendString(data);
USART1_SendString("\r\n");
}
void NB_IoT_ReceiveData(void)
{
USART1_SendString("AT+NSORF=0,20\r\n");
// Wait for response from NB-IoT module
// ...
// Read received data from NB-IoT module
// ...
}
```
其中,`USART1_Init()`函数初始化了STM32的USART1串口,用于与NB-IoT模块通信。`NB_IoT_Init()`函数初始化了NB-IoT模块。`NB_IoT_SendData()`函数发送数据到NB-IoT模块,`NB_IoT_ReceiveData()`函数接收数据从NB-IoT模块。
需要根据具体的NB-IoT模块和STM32微控制器进行修改和调整。
阅读全文
相关推荐
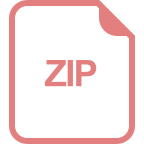
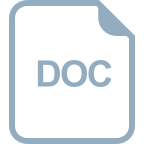

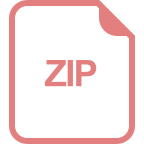
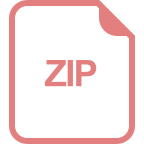
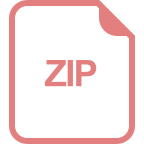
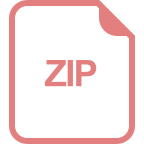
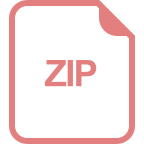
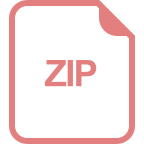
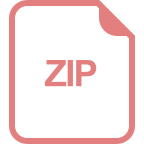
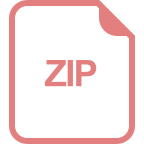
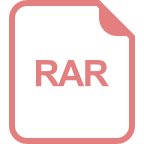
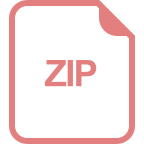
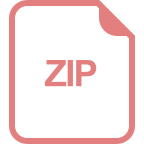
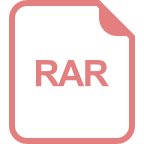
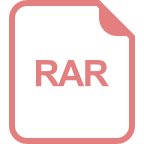