springboot 如何读取classpath文件
时间: 2023-09-11 17:04:59 浏览: 102
Spring Boot中有一个内置的Utility类ClassPathResource可以用于读取classpath下的文件。示例代码如下:
```java
import org.springframework.core.io.ClassPathResource;
import org.springframework.util.FileCopyUtils;
public class MyClass {
public static void main(String[] args) throws Exception {
ClassPathResource resource = new ClassPathResource("myFile.txt");
byte[] fileData = FileCopyUtils.copyToByteArray(resource.getInputStream());
String fileContent = new String(fileData, "UTF-8");
System.out.println(fileContent);
}
}
```
在上述示例中,我们读取了classpath下名为myFile.txt的文件,并将它的内容输出到控制台。如果文件是其他类型的文件,比如属性文件、XML文件、JSON文件等,读取方式会有所不同,但是基本的思路和使用方法是一样的。
相关问题
springboot读取resource文件
Spring Boot 通过使用 ResourceLoader 来读取 resource 文件。你可以使用 @Value 注解来读取文件中的值,也可以使用 ResourceLoader 的 getResource() 方法来读取文件。例如,下面的代码读取名为 "application.properties" 的文件:
```
@Autowired
private ResourceLoader resourceLoader;
Resource resource = resourceLoader.getResource("classpath:application.properties");
```
然后,可以使用 Resource 类的 getInputStream() 方法来读取文件的内容。
如果文件是yml格式,可以使用 @ConfigurationProperties 注解来读取。
```
@ConfigurationProperties(prefix = "file")
@PropertySource(value = "classpath:file.yml")
public class FileProperties{
private String path;
//getter setter
}
```
你可以在自己的代码中注入这个类,并使用它来读取文件中的值。
springboot读取text文件代码
您好!感谢您对ChitGPT的使用。以下是您所需的springboot读取text文件的代码示例。
首先,在您的Spring Boot项目中,您需要创建一个包含该文本文件的资源文件夹。您可以在src/main/resources下创建一个名为“data”的文件夹,并将该文件放置其中。
然后,您需要使用Spring的ResourceLoader类来加载该文件。下面是一个简单的示例代码:
```java
import org.springframework.core.io.Resource;
import org.springframework.core.io.ResourceLoader;
import org.springframework.stereotype.Component;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
@Component
public class TextFileReader {
private final ResourceLoader resourceLoader;
public TextFileReader(ResourceLoader resourceLoader) {
this.resourceLoader = resourceLoader;
}
public String readTextFile(String fileName) throws IOException {
Resource resource = resourceLoader.getResource("classpath:data/" + fileName);
InputStream inputStream = resource.getInputStream();
return convertInputStreamToString(inputStream);
}
private String convertInputStreamToString(InputStream inputStream) throws IOException {
StringBuilder resultStringBuilder = new StringBuilder();
try (BufferedReader br = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = br.readLine()) != null) {
resultStringBuilder.append(line).append("\n");
}
}
return resultStringBuilder.toString();
}
}
```
在该示例代码中,TextFileReader类注入了一个ResourceLoader,该对象提供了一种加载类路径资源的方法。readTextFile()方法接受一个文件名参数,并将其作为参数传递给ResourceLoader。然后,它使用getResource()方法获取该文件的资源,并从该资源中获取输入流。最后,它使用convertInputStreamToString()方法将输入流转换为字符串,并将其返回。
您可以在您的代码中使用这个TextFileReader类来读取文本文件。例如,如果要读取名为“example.txt”的文件,您可以执行以下代码:
```java
String fileContents = textFileReader.readTextFile("example.txt");
```
希望这个示例代码能够帮助您读取文本文件。如果您有任何其他问题,请随时问我!
阅读全文
相关推荐
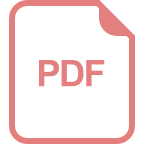
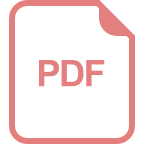
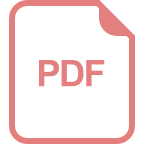
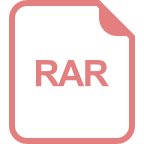
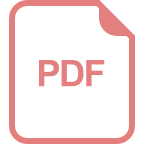
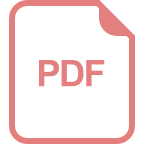





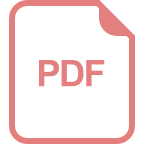




