stack(): argument 'tensors' (position 1) must be tuple of Tensors, not numpy.ndarray
时间: 2024-02-24 14:54:07 浏览: 60
这个错误通常表示你正在尝试将一个numpy数组作为参数传递给一个期望接收张量元组的函数。在PyTorch中,张量是指由torch.Tensor类表示的多维数组对象,而numpy数组是由NumPy库表示的多维数组对象。
要解决这个问题,你需要将numpy数组转换为PyTorch张量。你可以使用`torch.from_numpy()`函数将NumPy数组转换为PyTorch张量,然后再将其传递给期望接收张量元组的函数。
例如,假设你有一个名为`x_np`的NumPy数组,并且你想将其作为参数传递给`stack()`函数。你可以使用以下代码将`x_np`转换为PyTorch张量:
```
import torch
x = torch.from_numpy(x_np)
```
然后,你可以将`x`作为参数传递给`stack()`函数:
```
stacked = torch.stack((x,))
```
注意,`torch.stack()`函数期望传入一个张量元组,因此你需要使用双括号来将`x`包装在元组中。
相关问题
TypeError: stack(): argument 'tensors' (position 1) must be tuple of Tensors, not Tensor
This error occurs when the `stack()` function in PyTorch is called with a single tensor instead of a tuple of tensors.
To resolve this error, you need to pass a tuple of tensors to the `stack()` function instead of a single tensor. For example:
```
import torch
# Create two tensors
tensor1 = torch.tensor([1, 2, 3])
tensor2 = torch.tensor([4, 5, 6])
# Use stack() function to stack the tensors
stacked_tensors = torch.stack((tensor1, tensor2))
print(stacked_tensors)
```
This will output:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
Notice that we passed a tuple of tensors `(tensor1, tensor2)` to the `stack()` function.
AttributeError: 'numpy.ndarray' object has no attribute 'stack'
要解决"AttributeError: 'numpy.ndarray' object has no attribute 'stack'"错误,您可以尝试以下几个方法:
1. 检查您的代码中是否正确导入了numpy库。确保您使用的是正确的导入语句,例如`import numpy as np`。
2. 确保您的变量是一个numpy数组对象。检查您的代码中是否正确创建了一个numpy数组,并确认您正在对该数组使用正确的方法和属性。
3. 检查您的代码中是否存在拼写错误或语法错误。仔细检查您的代码,确保您使用了正确的方法名称和正确的参数。
如果您仍然遇到此错误,请提供更多的上下文和代码示例,以便我们能够更好地理解问题并提供更准确的解决方案。
相关推荐
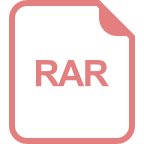
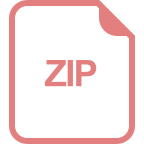












