现在我有一张图像A和图像A处理后得到的线段图,将图像和线段图分别输入resnet50中,得到两个1*512的图像特征,我该怎么输入自注意力模块计算得到自注意力损失以获得线段图像与原始 图像间的隐含关系,给出pytorch代码,并详细解释
时间: 2024-04-09 08:29:02 浏览: 12
要计算图像A和线段图像之间的隐含关系,可以使用自注意力模块,并计算自注意力损失。下面是使用PyTorch实现的示例代码:
```python
import torch
import torch.nn as nn
# 定义自注意力模块
class SelfAttentionModule(nn.Module):
def __init__(self, input_dim):
super(SelfAttentionModule, self).__init__()
self.input_dim = input_dim
self.query = nn.Linear(input_dim, input_dim)
self.key = nn.Linear(input_dim, input_dim)
self.value = nn.Linear(input_dim, input_dim)
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
# 计算query、key和value
query = self.query(x)
key = self.key(x)
value = self.value(x)
# 计算注意力分数
scores = torch.matmul(query, key.transpose(1, 2))
attention_weights = self.softmax(scores)
# 计算自注意力损失
attention_loss = torch.norm(attention_weights - torch.eye(x.size(1), device=x.device), p='fro')
# 计算注意力加权求和
attended_value = torch.matmul(attention_weights, value)
return attended_value, attention_loss
# 定义ResNet50模型
resnet = torchvision.models.resnet50(pretrained=True)
# 图像A和线段图像作为输入
image_A = torch.randn(1, 3, 224, 224) # 假设图像A为3通道的224x224图像
line_image = torch.randn(1, 3, 224, 224) # 假设线段图为3通道的224x224图像
# 分别将图像A和线段图像输入ResNet50
image_A_features = resnet(image_A)
line_image_features = resnet(line_image)
# 创建自注意力模块实例
attention_module = SelfAttentionModule(input_dim=512)
# 分别将图像特征输入自注意力模块
attended_image_A, attention_loss = attention_module(image_A_features)
attended_line_image, _ = attention_module(line_image_features)
# 打印自注意力损失
print("Attention Loss:", attention_loss)
```
上述代码首先定义了一个自注意力模块(SelfAttentionModule),其中包括查询(query)、键(key)和值(value)的线性变换层,以及一个softmax层用于计算注意力权重。在forward函数中,我们首先计算query、key和value,然后计算注意力分数。接着,我们使用softmax函数对注意力分数进行归一化,得到注意力权重。在计算自注意力损失时,我们使用了Frobenius范数(即矩阵的二范数)来度量注意力权重与单位矩阵之间的差异。最后,我们将注意力权重应用于值,并返回注意力加权求和的结果以及自注意力损失。
在示例代码中,我们使用了预训练的ResNet50模型来提取图像特征。然后,我们将图像特征分别输入自注意力模块,得到注意力加权求和后的图像特征(attended_image_A和attended_line_image),以及自注意力损失(attention_loss)。
需要注意的是,示例代码中的图像和线段图像的尺寸为224x224,并且假设输入的通道数为3。你可以根据实际情况修改代码中的图像尺寸和通道数。
相关推荐
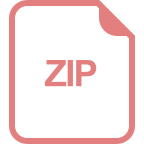
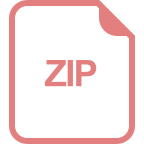














