package step1; import java.lang.Thread; public class MyThread extends Thread { private int num;//任务就是在子线程中计算num的阶乘 public MyThread() { this(0); } //constructor,创建实例的时候初始化参数 public MyThread(int num) { /begin your code here/ num=0; /end your code/ } @Override public void run() { //重写run方法,在子线程中想要执行的代码写在run方法中 int result = 1;//result保存计算出的结果 /begin your code here/ for(int i=1;i<=num;i++) { result*=i; } /end your code/ //直接输出结果 System.out.println(result); } }
时间: 2024-01-18 16:02:49 浏览: 194
好的,我来帮您补全代码。在构造函数中,应该将传入的参数赋值给成员变量num,而不是将成员变量num赋值为0。因此,需要修改如下:
```
public MyThread(int num) {
/begin your code here/
this.num=num;
/end your code/
}
```
在for循环中,需要将i从1循环到num,而不是从0循环到num-1,因此需要修改如下:
```
/begin your code here/
for(int i=1;i<=num;i++)
{
result*=i;
}
/end your code/
```
这样就完成了代码的补全。完整代码如下所示:
```
package step1;
import java.lang.Thread;
public class MyThread extends Thread {
private int num;//任务就是在子线程中计算num的阶乘
public MyThread() {
this(0);
}
//constructor,创建实例的时候初始化参数
public MyThread(int num) {
/begin your code here/
this.num=num;
/end your code/
}
@Override
public void run() {
//重写run方法,在子线程中想要执行的代码写在run方法中
int result = 1;//result保存计算出的结果
/begin your code here/
for(int i=1;i<=num;i++)
{
result*=i;
}
/end your code/
//直接输出结果
System.out.println(result);
}
}
```
阅读全文
相关推荐
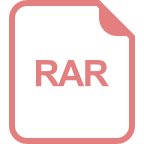
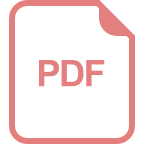
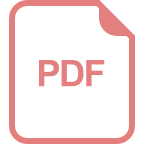












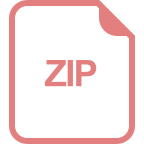