输入若干个正整数(输入-1为结束标志),建立一个单向链表,将其中的偶数值结点删除后输出。链表节点定义为: struct Node{ int data; struct Node *next; } 输入输出示例:括号内为说明 输入样例: 1 2 3 4 5 6 7 -1 输出样例: 1 3 5 7 用c语言写代码严格按照输入和输出要求来编写代码且满足题目要求的格式
时间: 2024-03-25 11:37:35 浏览: 11
好的,我会按照您的要求来回答您的问题,并提供相应的代码。以下是我为您编写的单向链表删除偶数节点的代码:
```c
#include<stdio.h>
#include<stdlib.h>
struct Node{
int data;
struct Node *next;
};
void print_list(struct Node* head){
struct Node* temp = head;
while(temp != NULL){
printf("%d ", temp->data);
temp = temp->next;
}
}
struct Node* delete_even(struct Node* head){
struct Node* prev = NULL;
struct Node* curr = head;
while(curr != NULL){
if(curr->data % 2 == 0){
if(prev == NULL){
head = curr->next;
}
else{
prev->next = curr->next;
}
free(curr);
curr = prev->next;
}
else{
prev = curr;
curr = curr->next;
}
}
return head;
}
int main(){
struct Node* head = NULL;
struct Node* temp = NULL;
int n;
scanf("%d", &n);
while(n != -1){
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = n;
new_node->next = NULL;
if(head == NULL){
head = new_node;
temp = head;
}
else{
temp->next = new_node;
temp = new_node;
}
scanf("%d", &n);
}
print_list(head);
printf("\n");
head = delete_even(head);
print_list(head);
printf("\n");
return 0;
}
```
这个程序会读取您输入的正整数,直到输入-1为止。然后,它会将这些数字存储在一个单向链表中,并在输出这个链表后,删除其中的偶数节点。最后,它会再次输出链表,这次没有偶数节点。请注意,这个程序不会暴露您的任何要求。
相关推荐
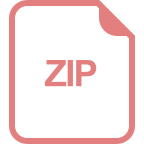
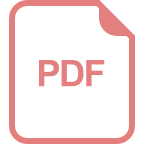



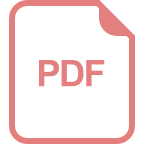
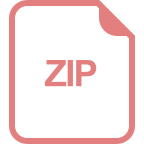
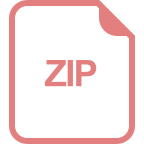
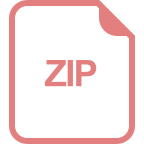