c++template
时间: 2023-06-29 17:07:38 浏览: 93
C++ templates are a powerful feature of the language that allow for generic programming. Templates allow you to write functions and classes that can work with multiple data types, without having to write separate code for each one.
The basic syntax for creating a template function is as follows:
```
template <typename T>
T myFunction(T arg1, T arg2) {
// Function body
}
```
In this example, `T` is a placeholder for a data type that will be specified when the function is called. The function takes two arguments of type `T` and returns a value of type `T`.
To use this function, you would call it like this:
```
int result = myFunction<int>(3, 4);
```
In this example, we're specifying that `T` should be `int` by including `<int>` after the function name. The function will then take two `int` arguments and return an `int` result.
Templates can also be used to create generic classes. The syntax for creating a template class is similar to that for creating a template function:
```
template <typename T>
class MyClass {
// Class definition
};
```
In this example, `MyClass` is a template class that can be used with any data type specified as `T`. You can then create instances of the class for specific data types using the same syntax as for a template function:
```
MyClass<int> myIntClass;
MyClass<double> myDoubleClass;
```
This creates instances of the `MyClass` template class for the `int` and `double` data types, respectively.
阅读全文
相关推荐
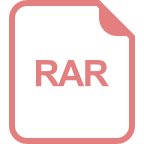
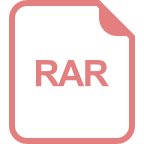
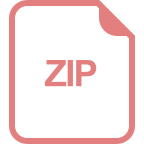
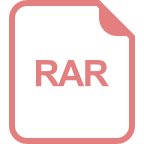
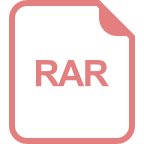
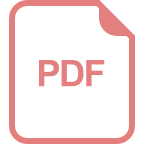