假设你是一家银行的开发人员,要求为帐户的类型定义对象,这些帐户是:活期帐户(Checking accout)储蓄存款帐户(Savings account)活期帐户具有以下特征帐户持有者的名字只能在创建帐户时指定初始余额(opening balance)必须在帐户创建时指定帐户创建时必须分配账户id号。活期帐户的账户id号范围是从100000到499999,每个活期帐户必须具有唯一的帐户id号。本实验不要求检查帐户号的上限。活期帐户持有者能够:定购支票簿检查帐户余额往帐户中加钱如果活期帐户里的资金充足,可以从中取钱储蓄存款帐户具有以下特征帐户持有者的名字只能在创建帐户时指定初始余额必须在帐户创建时指定储蓄存款帐户的账户id号范围是从500000到999999。本实验不要求检查帐户号的上限。帐户可以赚取利息。利息取决于帐户余额,如果余额大于1000,利息率是6%,否则是3%。储蓄存款帐户持有者能够:检查帐户余额往帐户中加钱如果活期帐户里的资金充足,可以从中取钱
时间: 2024-02-24 21:58:46 浏览: 357
这个问题涉及到面向对象编程的概念,需要定义两个类来实现活期帐户和储蓄存款帐户。下面是一个可能的代码实现:
```python
class Account:
def __init__(self, owner_name, opening_balance, account_id):
self.owner_name = owner_name
self.balance = opening_balance
self.account_id = account_id
def check_balance(self):
return self.balance
def deposit(self, amount):
self.balance += amount
class CheckingAccount(Account):
def __init__(self, owner_name, opening_balance, account_id):
super().__init__(owner_name, opening_balance, account_id)
if not (100000 <= account_id < 500000):
raise ValueError("Checking account ID must be between 100000 and 499999")
def order_checkbook(self):
# code to order a checkbook
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
raise ValueError("Insufficient funds")
class SavingsAccount(Account):
def __init__(self, owner_name, opening_balance, account_id):
super().__init__(owner_name, opening_balance, account_id)
if not (500000 <= account_id < 1000000):
raise ValueError("Savings account ID must be between 500000 and 999999")
def calculate_interest(self):
if self.balance >= 1000:
return self.balance * 0.06
else:
return self.balance * 0.03
```
这个实现中,`Account`是一个基类,包含了帐户共有的属性和方法。`CheckingAccount`和`SavingsAccount`是继承自`Account`的子类,分别实现了活期帐户和储蓄存款帐户的特定功能。例如,`CheckingAccount`实现了定购支票簿、取款等功能,而`SavingsAccount`实现了计算利息等功能。在创建活期帐户和储蓄存款帐户对象时,需要指定帐户持有者的名字、初始余额和账户id号。在活期帐户中,账户id号必须在100000到499999之间,而在储蓄存款帐户中,账户id号必须在500000到999999之间。如果创建对象时指定的账户id号不在合法范围内,会抛出`ValueError`异常。
阅读全文
相关推荐
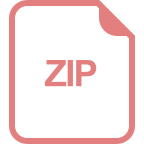
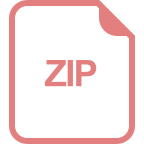
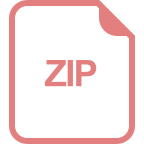















