python中删除csv文件的某一行
时间: 2023-09-07 09:15:44 浏览: 59
要删除CSV文件中的某一行数据,可以按照以下步骤进行操作:
1. 导入`csv`模块:
```python
import csv
```
2. 打开CSV文件并创建`csv.reader`对象:
```python
with open('file.csv', 'r') as file:
reader = csv.reader(file)
lines = list(reader) # 将所有行保存为列表
```
3. 根据需要删除某一行的数据:
```python
row_index = 2 # 要删除的行索引,假设为第3行
del lines[row_index] # 删除指定行的数据
```
4. 将修改后的数据写回CSV文件:
```python
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(lines) # 将所有行写入文件
```
这样就完成了对CSV文件某一行数据的删除。请将`file.csv`替换为你实际的文件路径,`row_index`替换为要删除的行索引。注意备份原始文件以防意外删除。
相关问题
python 实现删除csv文件某一行 源代码
import csv
def delete_row(csv_file_path, row_number):
with open(csv_file_path, 'r', newline='') as infile:
reader = csv.reader(infile)
rows = []
for index, row in enumerate(reader):
if index != row_number:
rows.append(row)
with open(csv_file_path, 'w', newline='') as outfile:
writer = csv.writer(outfile)
writer.writerows(rows)
# Example usage: delete row number 2 from file.csv
delete_row('file.csv', 2)
python删除csv文件某一行
你可以通过以下步骤删除CSV文件中的某一行:
1. 读取CSV文件为DataFrame对象
2. 使用`drop()`方法删除指定行
3. 将修改后的DataFrame保存为CSV文件
下面是一个示例代码:
```python
import pandas as pd
# 读取CSV文件为DataFrame对象
df = pd.read_csv('example.csv')
# 删除第3行
df = df.drop([2])
# 将修改后的DataFrame保存为CSV文件
df.to_csv('example.csv', index=False)
```
在上述代码中,我们使用`pd.read_csv()`方法读取CSV文件为DataFrame对象。然后,我们使用`drop()`方法删除第3行(即索引为2的行)。最后,我们使用`to_csv()`方法将修改后的DataFrame保存为CSV文件。`index=False`参数表示不保存行索引。
相关推荐
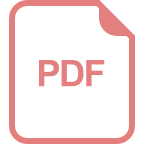
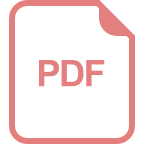











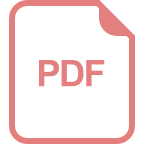