用python实现网站可视化
时间: 2024-06-09 14:04:53 浏览: 120
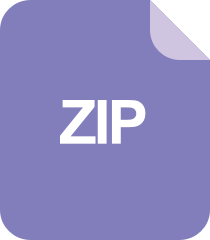
python制作Web可视化页面

要用 Python 实现网站可视化,有几种方法可以选择,下面介绍其中两种:
1. Flask
Flask 是一个 Python 的 Web 开发框架,可以用来快速搭建网站。通过 Flask,你可以将 Python 代码嵌入到 HTML 中,从而实现网站可视化。具体操作步骤如下:
- 安装 Flask:在终端中输入 `pip install Flask`。
- 编写 Python 代码:在 Python 文件中,通过 Flask 框架实现路由、视图函数等功能。例如:
```
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return 'Hello, World!'
@app.route('/about')
def about():
return render_template('about.html')
if __name__ == '__main__':
app.run()
```
- 编写 HTML 模板:在 `templates` 文件夹中,新建一个 HTML 文件,例如 `about.html`。在 HTML 文件中,可以使用 Flask 提供的模板语言,将 Python 变量、函数等嵌入到 HTML 中。例如:
```
<!DOCTYPE html>
<html>
<head>
<title>About</title>
</head>
<body>
<h1>About Us</h1>
<p>{{ message }}</p>
</body>
</html>
```
- 运行应用程序:在终端中输入 `python app.py`,然后在浏览器中访问 `http://localhost:5000/` 和 `http://localhost:5000/about`,查看效果。
2. Plotly Dash
Plotly Dash 是一个 Python 的 Web 应用框架,可以用来创建交互式数据可视化应用程序。通过 Plotly Dash,你可以使用 Python 代码实现网站可视化。具体操作步骤如下:
- 安装 Plotly Dash:在终端中输入 `pip install dash` 和 `pip install dash-bootstrap-components`。
- 编写 Python 代码:在 Python 文件中,通过 Dash 框架实现布局、回调函数等功能。例如:
```
import dash
import dash_bootstrap_components as dbc
import dash_core_components as dcc
import dash_html_components as html
from dash.dependencies import Input, Output
app = dash.Dash(external_stylesheets=[dbc.themes.BOOTSTRAP])
app.layout = html.Div([
dbc.NavbarSimple(
children=[
dbc.NavItem(dbc.NavLink("Home", href="#")),
dbc.NavItem(dbc.NavLink("About", href="#")),
],
brand="My Website",
brand_href="#",
color="primary",
dark=True,
),
html.Div([
html.H1("Welcome to my website!"),
dcc.Graph(id='example-graph', figure={
'data': [
{'x': [1, 2, 3], 'y': [4, 1, 2], 'type': 'bar', 'name': 'SF'},
{'x': [1, 2, 3], 'y': [2, 4, 5], 'type': 'bar', 'name': u'Montréal'},
],
'layout': {
'title': 'Dash Data Visualization'
}
})
], style={'textAlign': 'center'})
])
if __name__ == '__main__':
app.run_server()
```
- 运行应用程序:在终端中输入 `python app.py`,然后在浏览器中访问 `http://localhost:8050/`,查看效果。
以上是两种实现网站可视化的方法,你可以根据自己的需求选择适合自己的方式。
阅读全文
相关推荐
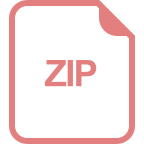
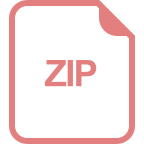
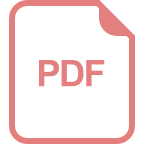
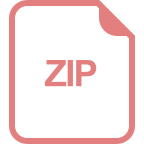
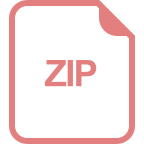
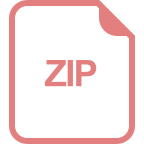
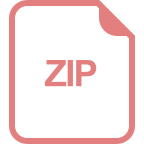
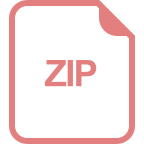
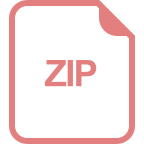
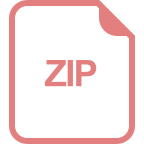
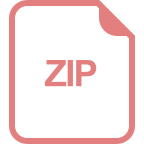
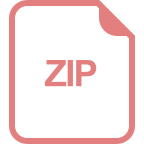
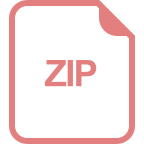
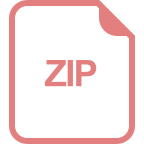
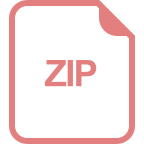