基于单链表的冒泡排序
时间: 2023-07-10 15:20:16 浏览: 47
好的,基于单链表的冒泡排序实现方法如下:
1. 首先,我们需要定义一个单链表的结构体,包括数据域和指向下一个节点的指针域。
```
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
```
2. 然后,我们需要实现一个函数来创建一个单链表,并初始化链表节点的值和指针。
```
ListNode* createList(vector<int>& nums) {
ListNode* head = new ListNode(0);
ListNode* cur = head;
for (int num : nums) {
cur->next = new ListNode(num);
cur = cur->next;
}
return head->next;
}
```
3. 接着,我们需要实现冒泡排序的函数。在单链表上实现冒泡排序需要注意节点的交换操作,需要交换节点的值,同时保持节点的指针不变。
```
ListNode* bubbleSortList(ListNode* head) {
if (!head || !head->next) {
return head;
}
ListNode* dummy = new ListNode(0);
dummy->next = head;
ListNode* pre = dummy;
ListNode* cur = head;
bool flag = true;
while (flag) {
flag = false;
while (cur->next) {
if (cur->val > cur->next->val) {
ListNode* tmp = cur->next;
cur->next = cur->next->next;
tmp->next = cur;
pre->next = tmp;
pre = tmp;
flag = true;
} else {
pre = cur;
cur = cur->next;
}
}
pre = dummy;
cur = dummy->next;
}
return dummy->next;
}
```
4. 最后,我们可以测试一下冒泡排序的函数。
```
int main() {
vector<int> nums = {4, 2, 1, 3};
ListNode* head = createList(nums);
ListNode* sortedList = bubbleSortList(head);
while (sortedList) {
cout << sortedList->val << " ";
sortedList = sortedList->next;
}
cout << endl;
return 0;
}
```
输出结果为:1 2 3 4。
希望这个实现方法能够帮到你!
相关推荐
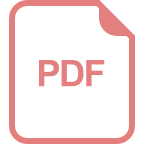
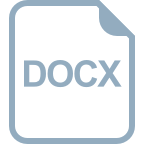
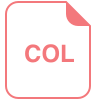
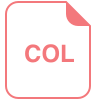
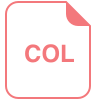
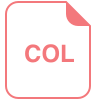
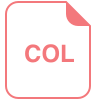









