请编写程序,用户输入姓名和分数,将输入的记录加进文本文件, 并显示当前的最高分的姓名。(文本文件内容如下)。 王益诚,91 李裕,89 张洪源,79 马柏源,77 叶维鑫,68 熊杰,61 和金贵,88 杨钊,62 王林刚,92 彭林,84
时间: 2024-03-17 19:39:51 浏览: 86
好的,这里提供一种 Python 的实现方式:
```python
# 打开文本文件,读取内容
with open('scores.txt', 'r') as f:
lines = f.readlines()
# 解析每行记录,生成字典,存入列表
records = []
for line in lines:
name, score = line.strip().split(',')
record = {'name': name, 'score': int(score)}
records.append(record)
# 获取用户输入,添加到列表
name = input('请输入姓名:')
score = int(input('请输入分数:'))
record = {'name': name, 'score': score}
records.append(record)
# 将列表按分数降序排序
records = sorted(records, key=lambda x: x['score'], reverse=True)
# 将排序后的记录写回文本文件
with open('scores.txt', 'w') as f:
for record in records:
line = f"{record['name']},{record['score']}\n"
f.write(line)
# 显示当前最高分的姓名
highest = records[0]['name']
print(f'当前最高分的姓名为:{highest}')
```
需要注意的是,文件中的中文逗号和空格都需要正确处理。另外,此程序假设所有输入都是合法的,没有做输入校验。
阅读全文
相关推荐
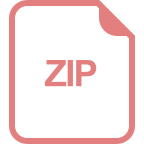











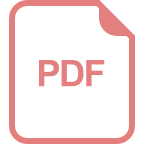
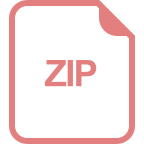