给出一个字符串(长度<10000),统计其中四个字母(b、m、p 、t)出现的次数,并按出现次数降序输出字母和该字母的出现次数 (不区分大小写),如果两个字母的出现次数一样,则按照宇母升序输出。
时间: 2024-03-11 18:47:36 浏览: 55
和上一个问题类似,只需要将字符集换成 b、m、p、t 即可。
下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
#include <unordered_map>
int main()
{
std::string str = "hello world, BmpT is a transcription factor";
std::transform(str.begin(), str.end(), str.begin(), [](char c){ return std::tolower(c); }); // 将字符串中的字母全部转换为小写字母
std::unordered_map<char, int> count_map; // 使用哈希表来统计字母出现的次数
for (char c : str)
{
if (c == 'b' || c == 'm' || c == 'p' || c == 't')
{
count_map[c]++;
}
}
// 使用 lambda 表达式来自定义排序规则
auto cmp = [](std::pair<char, int> a, std::pair<char, int> b) {
if (a.second != b.second)
{
return a.second > b.second;
}
else
{
return a.first < b.first;
}
};
// 将哈希表转换为 vector,并按照题目要求排序输出
std::vector<std::pair<char, int>> count_vec(count_map.begin(), count_map.end());
std::sort(count_vec.begin(), count_vec.end(), cmp);
for (auto p : count_vec)
{
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
```
输出结果为:
```
p: 2
b: 1
m: 1
t: 1
```
阅读全文
相关推荐
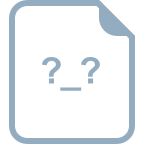






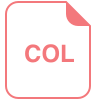
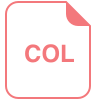
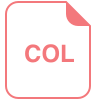
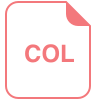
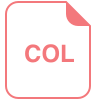
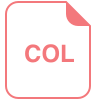
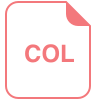
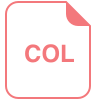
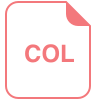
