遗传算法python实例
时间: 2023-10-26 10:08:10 浏览: 11
以下是一个简单的遗传算法 Python 实现示例:
```python
import random
# 遗传算法参数
POPULATION_SIZE = 100
PARENTS_SIZE = 50
GENERATIONS = 100
MUTATION_RATE = 0.05
# 目标函数:计算x的平方根
def fitness(x):
return x ** 0.5
# 随机生成种群
def generate_population(size):
population = []
for i in range(size):
individual = {'x': random.uniform(0, 100)}
individual['fitness'] = fitness(individual['x'])
population.append(individual)
return population
# 选择父母
def select_parents(population, size):
parents = []
for i in range(size):
candidates = random.sample(population, 3)
candidates.sort(key=lambda x: x['fitness'], reverse=True)
parents.append(candidates[0])
return parents
# 交叉
def crossover(parents):
offspring = []
for i in range(len(parents)):
parent1 = parents[i]
parent2 = parents[(i + 1) % len(parents)]
child = {'x': (parent1['x'] + parent2['x']) / 2.0}
child['fitness'] = fitness(child['x'])
offspring.append(child)
return offspring
# 变异
def mutate(individual):
if random.random() < MUTATION_RATE:
individual['x'] = random.uniform(0, 100)
individual['fitness'] = fitness(individual['x'])
# 遗传算法主程序
def run_ga():
population = generate_population(POPULATION_SIZE)
for i in range(GENERATIONS):
parents = select_parents(population, PARENTS_SIZE)
offspring = crossover(parents)
for child in offspring:
mutate(child)
population = parents + offspring
population.sort(key=lambda x: x['fitness'], reverse=True)
population = population[:POPULATION_SIZE]
print('Generation %d: Best fitness = %.2f' % (i, population[0]['fitness']))
return population[0]['x']
# 运行遗传算法
best_x = run_ga()
print('Best solution: x = %.2f, fitness = %.2f' % (best_x, fitness(best_x)))
```
该遗传算法的目标是最大化函数`fitness(x) = x ** 0.5`,即计算`x`的平方根。在每一代中,它随机生成一个种群,然后选择父母进行交叉产生下一代,并使用变异操作添加随机性。该算法在每一代中保留最适应的个体,并在每一代结束时输出最佳适应度。在最后一代中,它输出最佳解决方案(`x`的值)和相应的适应度。
阅读全文
相关推荐
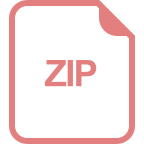
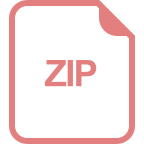
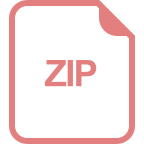
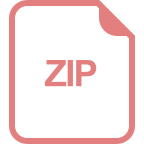
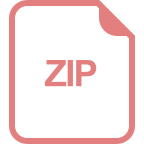
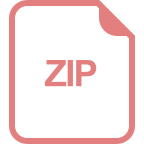