调用mediapipe opencv手势识别1到10,根据对应手势显示出d盘里的图片 给出代码
时间: 2024-03-01 22:53:47 浏览: 173
以下是一个使用mediapipe和OpenCV进行手势识别并显示对应图片的示例代码:
```
import cv2
import os
import mediapipe as mp
# 设置图像路径
image_dir = 'D:/images/'
# 加载图像
def load_image(image_path):
img = cv2.imread(image_path)
img = cv2.resize(img, (400, 400))
return img
# 加载所有图像
images = []
for i in range(1, 11):
image_path = os.path.join(image_dir, '{}.jpg'.format(i))
images.append(load_image(image_path))
# 加载MediaPipe Handpose模型
mp_hands = mp.solutions.hands
hands = mp_hands.Hands(static_image_mode=True, max_num_hands=1, min_detection_confidence=0.5)
# 加载MediaPipe Handpose绘图工具
mp_drawing = mp.solutions.drawing_utils
# 加载OpenCV
cap = cv2.VideoCapture(0)
while True:
# 读取图像
success, image = cap.read()
if not success:
break
# 转换为RGB格式
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# 调用MediaPipe Handpose模型进行手势识别
results = hands.process(image)
# 绘制手势
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
mp_drawing.draw_landmarks(image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
# 获取手势
thumb_tip = hand_landmarks.landmark[mp_hands.HandLandmark.THUMB_TIP]
index_tip = hand_landmarks.landmark[mp_hands.HandLandmark.INDEX_FINGER_TIP]
middle_tip = hand_landmarks.landmark[mp_hands.HandLandmark.MIDDLE_FINGER_TIP]
ring_tip = hand_landmarks.landmark[mp_hands.HandLandmark.RING_FINGER_TIP]
little_tip = hand_landmarks.landmark[mp_hands.HandLandmark.PINKY_TIP]
# 根据手势显示对应图片
if thumb_tip.y < index_tip.y and thumb_tip.y < middle_tip.y and thumb_tip.y < ring_tip.y and thumb_tip.y < little_tip.y:
cv2.imshow('Image', images[0])
elif thumb_tip.y > index_tip.y and thumb_tip.y > middle_tip.y and thumb_tip.y > ring_tip.y and thumb_tip.y > little_tip.y:
cv2.imshow('Image', images[10])
elif index_tip.y < thumb_tip.y and middle_tip.y < thumb_tip.y and ring_tip.y < thumb_tip.y and little_tip.y < thumb_tip.y:
cv2.imshow('Image', images[1])
elif thumb_tip.y < index_tip.y and middle_tip.y < index_tip.y and ring_tip.y < index_tip.y and little_tip.y < index_tip.y:
cv2.imshow('Image', images[2])
elif thumb_tip.y < index_tip.y and thumb_tip.y < middle_tip.y and ring_tip.y < middle_tip.y and little_tip.y < middle_tip.y:
cv2.imshow('Image', images[3])
elif thumb_tip.y < index_tip.y and thumb_tip.y < middle_tip.y and thumb_tip.y < ring_tip.y and little_tip.y < ring_tip.y:
cv2.imshow('Image', images[4])
elif thumb_tip.y > index_tip.y and middle_tip.y < index_tip.y and ring_tip.y < index_tip.y and little_tip.y < index_tip.y:
cv2.imshow('Image', images[5])
elif thumb_tip.y > index_tip.y and thumb_tip.y > middle_tip.y and ring_tip.y < little_tip.y:
cv2.imshow('Image', images[6])
elif thumb_tip.y < index_tip.y and middle_tip.y < index_tip.y and ring_tip.y > index_tip.y and little_tip.y > index_tip.y:
cv2.imshow('Image', images[7])
elif thumb_tip.y < index_tip.y and middle_tip.y < index_tip.y and ring_tip.y < index_tip.y and little_tip.y > index_tip.y:
cv2.imshow('Image', images[8])
else:
cv2.imshow('Image', images[9])
else:
cv2.imshow('Image', image)
# 等待按键事件
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
在这个示例代码中,我们首先加载了所有手势对应的图片,并加载了MediaPipe Handpose模型和绘图工具。然后通过OpenCV获取视频流,并对每一帧图像进行手势识别和对应图片的显示。在根据手势显示对应图片的部分,我们通过判断各关键点的位置关系来判断手势类型,并显示对应的图片。
需要注意的是,这个示例代码只是一个简单的示例,实际的手势识别还需要考虑更多因素,例如光照、背景等。
阅读全文
相关推荐














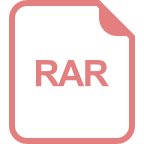


