Write a Python function named "validate_password" that takes a string as input and checks if it is a valid password. A valid password should meet the following criteria: • It must be at least 8 characters long. • It must contain at least one uppercase letter, one lowercase letter, and one digit.use python
时间: 2024-03-10 10:45:49 浏览: 18
Sure, here is the code for the "validate_password" function in Python:
```python
def validate_password(password):
if len(password) < 8:
return False
has_uppercase = False
has_lowercase = False
has_digit = False
for char in password:
if char.isupper():
has_uppercase = True
elif char.islower():
has_lowercase = True
elif char.isdigit():
has_digit = True
if has_uppercase and has_lowercase and has_digit:
return True
else:
return False
```
This function takes a string "password" as input and first checks if its length is less than 8 characters. If it is, the function returns False.
Next, the function initializes three boolean variables - "has_uppercase", "has_lowercase", and "has_digit" - to False. It then loops through each character in the password string and checks if it is an uppercase letter, lowercase letter, or digit. If a character of each type is found, the corresponding boolean variable is set to True.
Finally, the function checks if all three boolean variables are True. If they are, the function returns True to indicate that the password is valid. Otherwise, it returns False.
相关推荐
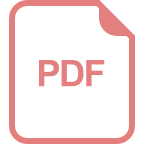
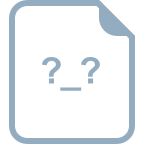















