openpgp加密工具_使用OpenPGP处理前端加密
时间: 2023-12-25 14:05:33 浏览: 121
OpenPGP是一种加密和签名标准,常用于保护电子邮件的内容。在前端加密中,可以使用OpenPGP库来实现数据的加密和解密。以下是在前端使用OpenPGP库进行加密的示例代码:
1. 安装OpenPGP库
可以使用npm包管理器安装OpenPGP库:
```
npm install --save openpgp
```
2. 生成公钥和私钥
在前端中使用OpenPGP时,需要生成公钥和私钥。可以使用以下代码生成密钥对:
```javascript
const openpgp = require('openpgp');
// 生成密钥对
openpgp.generateKey({
userIds: [{ name: 'John Doe', email: 'johndoe@example.com' }],
curve: 'ed25519',
passphrase: 'supersecret'
}).then((keys) => {
const publicKeyArmored = keys.publicKeyArmored;
const privateKeyArmored = keys.privateKeyArmored;
});
```
3. 加密数据
生成密钥对后,可以使用公钥加密数据。以下是使用OpenPGP库加密数据的示例代码:
```javascript
const openpgp = require('openpgp');
// 加载公钥
const publicKey = `-----BEGIN PGP PUBLIC KEY BLOCK-----
...
-----END PGP PUBLIC KEY BLOCK-----`;
// 加密数据
const plaintext = 'Hello, world!';
openpgp.encrypt({
message: openpgp.message.fromText(plaintext),
publicKeys: openpgp.key.readArmored(publicKey).keys
}).then((ciphertext) => {
const encrypted = ciphertext.data;
});
```
4. 解密数据
使用私钥可以解密数据。以下是使用OpenPGP库解密数据的示例代码:
```javascript
const openpgp = require('openpgp');
// 加载私钥
const privateKey = `-----BEGIN PGP PRIVATE KEY BLOCK-----
...
-----END PGP PRIVATE KEY BLOCK-----`;
// 解密数据
const encrypted = '...';
openpgp.decrypt({
message: openpgp.message.readArmored(encrypted),
privateKeys: openpgp.key.readArmored(privateKey).keys,
passphrase: 'supersecret'
}).then((plaintext) => {
const decrypted = plaintext.data;
});
```
使用OpenPGP库可以很方便地实现前端加密和解密。但需要注意的是,私钥需要保护好,以免泄露。
阅读全文
相关推荐
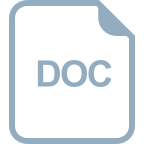
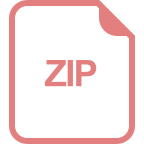
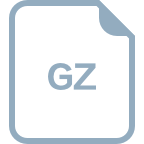
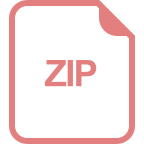
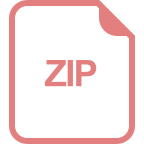
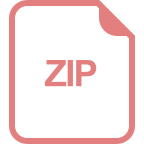
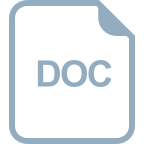
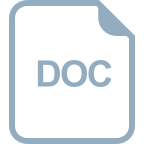
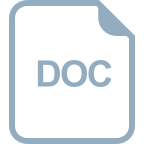
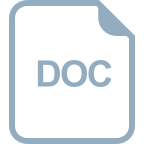
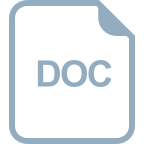
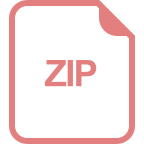
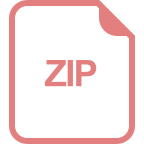
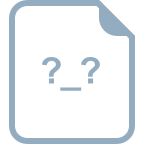
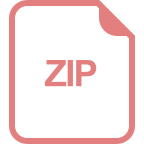