Java实现使用ServerSocket类和Socket类以及InetAddress类编写一个GUI实现一个简单的聊天程序
时间: 2024-02-11 10:04:28 浏览: 92
好的,我来回答你的问题。
首先,我们需要了解一下 ServerSocket、Socket 和 InetAddress 这三个类的作用:
- ServerSocket:用于监听客户端的连接请求,并创建对应的 Socket 对象进行通信。
- Socket:用于与服务器建立连接并进行通信。
- InetAddress:用于表示 IP 地址。
接下来,我们可以开始编写聊天程序了。下面是一个简单的 Java 聊天程序的代码:
1. 服务器端代码:
```java
import java.io.*;
import java.net.*;
import javax.swing.*;
public class Server extends JFrame {
private JTextField userText;
private JTextArea chatWindow;
private ObjectOutputStream output;
private ObjectInputStream input;
private ServerSocket server;
private Socket connection;
// 构造方法
public Server() {
super("Server");
userText = new JTextField();
userText.setEditable(false);
userText.addActionListener(event -> sendMessage(event.getActionCommand()));
add(userText, BorderLayout.NORTH);
chatWindow = new JTextArea();
add(new JScrollPane(chatWindow));
setSize(300, 150);
setVisible(true);
}
// 启动服务器
public void startRunning() {
try {
server = new ServerSocket(7777, 100);
while (true) {
try {
waitForConnection();
setupStreams();
whileChatting();
} catch (EOFException eofException) {
showMessage("\nServer ended the connection!");
} finally {
closeCrap();
}
}
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
// 等待连接
private void waitForConnection() throws IOException {
showMessage("Waiting for someone to connect...\n");
connection = server.accept();
showMessage("Now connected to " + connection.getInetAddress().getHostName());
}
// 设置流
private void setupStreams() throws IOException {
output = new ObjectOutputStream(connection.getOutputStream());
output.flush();
input = new ObjectInputStream(connection.getInputStream());
showMessage("\nStreams are now setup!\n");
}
// 聊天
private void whileChatting() throws IOException {
String message = "You are now connected!";
sendMessage(message);
ableToType(true);
do {
try {
message = (String) input.readObject();
showMessage("\n" + message);
} catch (ClassNotFoundException classNotFoundException) {
showMessage("\nUnknown object type received!");
}
} while (!message.equals("CLIENT - END"));
}
// 关闭连接
private void closeCrap() {
showMessage("\nClosing connections...\n");
ableToType(false);
try {
output.close();
input.close();
connection.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
// 发送消息
private void sendMessage(String message) {
try {
output.writeObject("SERVER - " + message);
output.flush();
showMessage("\nSERVER - " + message);
} catch (IOException ioException) {
chatWindow.append("\nERROR: Cannot send message!");
}
}
// 更新聊天窗口
private void showMessage(String message) {
SwingUtilities.invokeLater(() -> chatWindow.append(message));
}
// 是否可以输入
private void ableToType(boolean tof) {
SwingUtilities.invokeLater(() -> userText.setEditable(tof));
}
}
```
2. 客户端代码:
```java
import java.io.*;
import java.net.*;
import javax.swing.*;
public class Client extends JFrame {
private JTextField userText;
private JTextArea chatWindow;
private ObjectOutputStream output;
private ObjectInputStream input;
private String message = "";
private String serverIP;
private Socket connection;
// 构造方法
public Client(String host) {
super("Client");
serverIP = host;
userText = new JTextField();
userText.setEditable(false);
userText.addActionListener(event -> sendMessage(event.getActionCommand()));
add(userText, BorderLayout.NORTH);
chatWindow = new JTextArea();
add(new JScrollPane(chatWindow));
setSize(300, 150);
setVisible(true);
}
// 连接到服务器
public void startRunning() {
try {
connectToServer();
setupStreams();
whileChatting();
} catch (EOFException eofException) {
showMessage("\nClient terminated the connection!");
} catch (IOException ioException) {
ioException.printStackTrace();
} finally {
closeCrap();
}
}
// 连接到服务器
private void connectToServer() throws IOException {
showMessage("Attempting connection...\n");
connection = new Socket(InetAddress.getByName(serverIP), 7777);
showMessage("Connected to: " + connection.getInetAddress().getHostName());
}
// 设置流
private void setupStreams() throws IOException {
output = new ObjectOutputStream(connection.getOutputStream());
output.flush();
input = new ObjectInputStream(connection.getInputStream());
showMessage("\nStreams are now setup!\n");
}
// 聊天
private void whileChatting() throws IOException {
ableToType(true);
do {
try {
message = (String) input.readObject();
showMessage("\n" + message);
} catch (ClassNotFoundException classNotFoundException) {
showMessage("\nUnknown object type received!");
}
} while (!message.equals("SERVER - END"));
}
// 关闭连接
private void closeCrap() {
showMessage("\nClosing connections...\n");
ableToType(false);
try {
output.close();
input.close();
connection.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
// 发送消息
private void sendMessage(String message) {
try {
output.writeObject("CLIENT - " + message);
output.flush();
showMessage("\nCLIENT - " + message);
} catch (IOException ioException) {
chatWindow.append("\nERROR: Cannot send message!");
}
}
// 更新聊天窗口
private void showMessage(String message) {
SwingUtilities.invokeLater(() -> chatWindow.append(message));
}
// 是否可以输入
private void ableToType(boolean tof) {
SwingUtilities.invokeLater(() -> userText.setEditable(tof));
}
}
```
以上就是一个使用 ServerSocket 类和 Socket 类以及 InetAddress 类编写的简单聊天程序的代码。
阅读全文
相关推荐



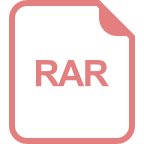

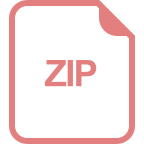










