用c语言编写一个程序实现链队列的各种基本运算,并在此基础上设计一个主程序,我成如下功能: (1)初始化并建立链队列; (2)入队操作;(3)出队操作;(4) 遍历队列; (5)判断队空操作; (6),取队头和队尾操作 (7)求队长
时间: 2024-10-22 09:09:56 浏览: 57
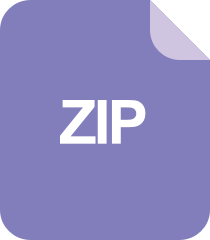
C语言必背15个经典程序_15道C语言编程_c语言必备十五个经典程序_
在C语言中,我们可以使用结构体和指针来实现链队列的数据结构。首先,我们需要定义一个节点结构体,包含数据域和指向下一个节点的指针。接下来,我们分别实现各个功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链队列
void createQueue(Node** head_ref) {
*head_ref = NULL;
}
// 入队操作 - 在队尾添加元素
void enqueue(Node** head_ref, int item) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = item;
newNode->next = NULL;
if (*head_ref == NULL) {
*head_ref = newNode;
} else {
Node* last = *head_ref;
while (last->next != NULL) {
last = last->next;
}
last->next = newNode;
}
}
// 出队操作 - 删除队头元素
int dequeue(Node** head_ref) {
if (*head_ref == NULL) {
printf("Queue is empty.\n");
return -1; // 返回特殊值表示队空
}
int removedItem = (*head_ref)->data;
Node* temp = *head_ref;
*head_ref = (*head_ref)->next;
free(temp); // 释放内存
return removedItem;
}
// 遍历队列
void traverseQueue(Node* head) {
if (head == NULL) {
printf("Queue is empty.\n");
return;
}
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 判断队是否为空
int isEmpty(Node* head) {
return head == NULL;
}
// 取队头操作
int frontOfQueue(Node* head) {
if (isEmpty(head)) {
printf("Queue is empty.\n");
return -1;
}
return head->data;
}
// 取队尾操作
int rearOfQueue(Node* head) {
if (isEmpty(head)) {
printf("Queue is empty.\n");
return -1;
}
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
return current->data;
}
// 求队长 - 队首元素的位置,假设队列非空
int getQueueLength(Node* head) {
int length = 0;
Node* current = head;
while (current != NULL) {
length++;
current = current->next;
}
return length;
}
int main() {
Node* queueHead = NULL;
// 初始化并建立链队列
createQueue(&queueHead);
// 示例操作
enqueue(&queueHead, 1);
enqueue(&queueHead, 2);
enqueue(&queueHead, 3);
printf("Front of queue: %d\n", frontOfQueue(queueHead)); // 应该输出1
printf("Rear of queue: %d\n", rearOfQueue(queueHead)); // 应该输出3
traverseQueue(queueHead); // 遍历打印:1 2 3
printf("Is queue empty? %s\n", isEmpty(queueHead) ? "Yes" : "No"); // 输出No
dequeue(&queueHead); // 出队1,队列变为2, 3
printf("Queue after dequeue: ");
traverseQueue(queueHead); // 打印:2 3
printf("Length of the queue: %d\n", getQueueLength(queueHead)); // 输出2
return 0;
}
```
这个示例展示了如何在C语言中实现链队列的基本操作。记得在实际运行此代码前,需要在合适的位置处理可能出现的内存分配失败等错误情况。
阅读全文
相关推荐
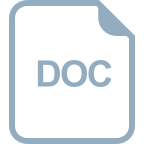
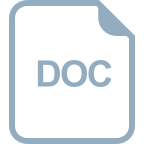

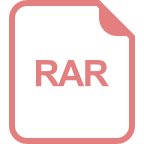
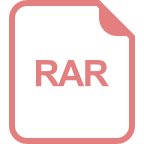
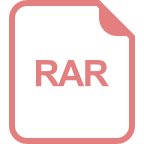
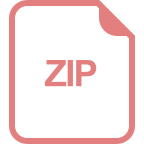
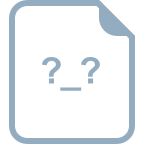
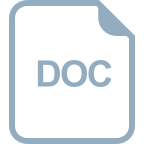
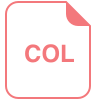
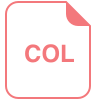
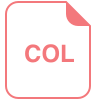
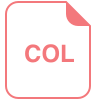
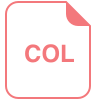
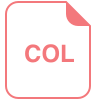
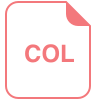
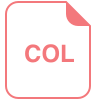