python凯撒密码加解密
时间: 2023-10-13 22:18:30 浏览: 114
凯撒密码是一种简单的加密算法,它将明文中的每个字母按照一个固定的偏移量进行移位,从而得到密文。加密和解密的偏移量必须相同。
以下是Python实现凯撒密码的加解密代码:
```python
def caesar_encrypt(text, shift):
"""
凯撒密码加密函数
:param text: 明文
:param shift: 偏移量
:return: 密文
"""
result = ""
# 遍历明文中的每个字符
for i in range(len(text)):
char = text[i]
# 如果是大写字母,将其转换为ASCII码,加上偏移量,然后再转换回字符
if char.isupper():
result += chr((ord(char) + shift - 65) % 26 + 65)
# 如果是小写字母,同理
elif char.islower():
result += chr((ord(char) + shift - 97) % 26 + 97)
else:
result += char
return result
def caesar_decrypt(text, shift):
"""
凯撒密码解密函数
:param text: 密文
:param shift: 偏移量
:return: 明文
"""
result = ""
# 遍历密文中的每个字符
for i in range(len(text)):
char = text[i]
# 如果是大写字母,将其转换为ASCII码,减去偏移量,然后再转换回字符
if char.isupper():
result += chr((ord(char) - shift - 65) % 26 + 65)
# 如果是小写字母,同理
elif char.islower():
result += chr((ord(char) - shift - 97) % 26 + 97)
else:
result += char
return result
# 测试
text = "Hello, World!"
shift = 3
encrypted = caesar_encrypt(text, shift)
decrypted = caesar_decrypt(encrypted, shift)
print("明文:", text)
print("密文:", encrypted)
print("解密后的明文:", decrypted)
```
输出结果:
```
明文: Hello, World!
密文: Khoor, Zruog!
解密后的明文: Hello, World!
```
注意,在加密和解密时,偏移量必须相同。
阅读全文
相关推荐
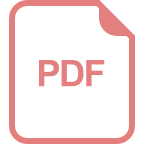
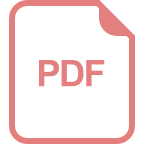
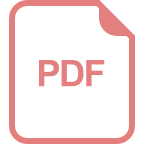

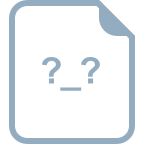
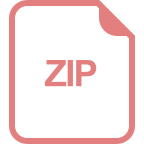



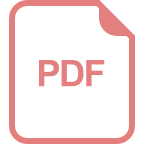
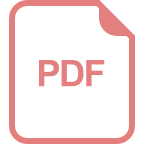
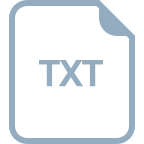
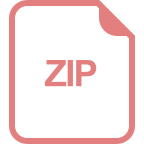
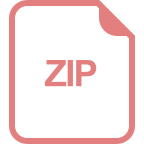