如果用栈存放MPMC问题,请给出解决该问题的伪代码
时间: 2023-08-21 09:05:09 浏览: 33
解决MPMC问题的一种方法是使用栈(Stack)数据结构,以下是该算法的伪代码:
```
// 定义栈节点
struct Node {
int data;
Node* next;
};
// 定义栈
struct Stack {
Node* head;
void push(int data) {
Node* new_node = new Node();
new_node->data = data;
do {
new_node->next = head;
} while (!atomic_compare_exchange_weak(&head, &new_node->next, new_node));
}
int pop() {
Node* old_head;
do {
old_head = head;
if (old_head == nullptr) {
return -1; // 栈为空,返回-1
}
} while (!atomic_compare_exchange_weak(&head, &old_head, old_head->next));
int data = old_head->data;
delete old_head;
return data;
}
};
// 定义多个生产者线程和多个消费者线程
Stack stack;
atomic<int> count;
// 生产者线程
void producer_thread(int id) {
while (true) {
int data = produce_data();
while (count >= CAPACITY); // 队列已满,自旋等待
stack.push(data);
count++;
}
}
// 消费者线程
void consumer_thread(int id) {
while (true) {
while (count <= 0); // 队列已空,自旋等待
int data = stack.pop();
if (data != -1) {
consume_data(data);
count--;
}
}
}
```
其中,`atomic_compare_exchange_weak` 是一个原子操作,它可以保证在多线程环境下对栈进行原子操作。在 push 操作中,使用了一个自旋循环来保证 CAS(Compare-And-Swap)操作成功,以避免在多线程环境下发生竞争。在 pop 操作中,同样使用了自旋循环和 CAS 操作,以保证线程安全。
需要注意的是,这里的栈是有界的(即有容量限制),因此在生产者线程中需要判断栈是否已满,而在消费者线程中需要判断栈是否已空。同时,为了避免消费者线程在栈为空时进行 pop 操作,pop 操作需要返回一个特殊值(例如 -1),表示栈为空。
此外,这个算法也可以使用其他数据结构来实现,例如循环缓冲区(Circular Buffer)等。
相关推荐
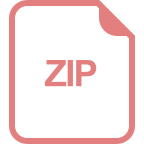
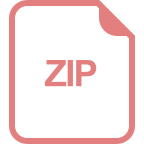



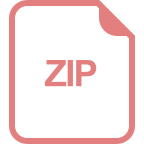
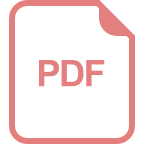
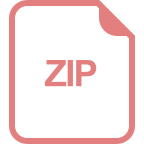
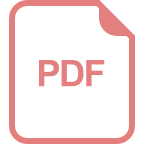
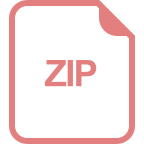
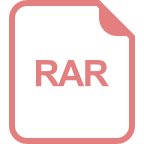
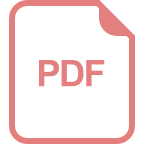