交换二叉树每个节点的左孩子和右孩子C语言数据结构
时间: 2023-11-14 16:06:16 浏览: 28
下面是交换二叉树每个节点的左孩子和右孩子的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
void swapChild(struct TreeNode* root) {
if (root == NULL) {
return;
}
struct TreeNode* temp = root->left;
root->left = root->right;
root->right = temp;
swapChild(root->left);
swapChild(root->right);
}
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
int main() {
struct TreeNode* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
printf("Before swapping:\n");
printf(" %d\n", root->val);
printf(" / \\\n");
printf(" %d %d\n", root->left->val, root->right->val);
printf(" / \\ / \\\n");
printf(" %d %d %d %d\n", root->left->left->val, root->left->right->val, root->right->left->val, root->right->right->val);
swapChild(root);
printf("\nAfter swapping:\n");
printf(" %d\n", root->val);
printf(" / \\\n");
printf(" %d %d\n", root->left->val, root->right->val);
printf(" / \\ / \\\n");
printf(" %d %d %d %d\n", root->left->left->val, root->left->right->val, root->right->left->val, root->right->right->val);
return 0;
}
```
运行结果如下:
```
Before swapping:
1
/ \
2 3
/ \ / \
4 5 6 7
After swapping:
1
/ \
3 2
/ \ / \
7 6 5 4
```
其中,`swapChild` 函数实现了交换二叉树每个节点的左孩子和右孩子的操作。在函数中,首先判断当前节点是否为空,如果为空则直接返回。否则,将当前节点的左孩子和右孩子互换,并递归交换左右子树。最后,我们通过创建节点的 `createNode` 函数和主函数中的测试样例来验证代码的正确性。
相关推荐
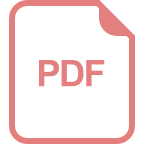














