nodejs mysql 做一个图书管理系统
时间: 2024-01-31 08:04:08 浏览: 88
作为AI语言模型,我可以为您提供一些关于如何使用Node.js和MySQL创建图书管理系统的指导。
1. 创建数据库和表
首先,您需要创建一个MySQL数据库和一个名为“books”的表,该表将存储所有图书的信息。在MySQL控制台中,可以使用以下命令来创建数据库和表:
```
CREATE DATABASE library;
USE library;
CREATE TABLE books (
id INT NOT NULL AUTO_INCREMENT,
title VARCHAR(255) NOT NULL,
author VARCHAR(255) NOT NULL,
year INT NOT NULL,
PRIMARY KEY (id)
);
```
2. 安装依赖库
您需要安装以下Node.js模块来连接和操作MySQL数据库:
```
npm install mysql --save
npm install express --save
```
3. 创建Express应用程序
在您的项目文件夹中创建一个名为“app.js”的文件,并编写以下代码:
```
const express = require('express');
const mysql = require('mysql');
const app = express();
const port = 3000;
const db = mysql.createConnection({
host: 'localhost',
user: 'root',
password: '',
database: 'library'
});
db.connect((err) => {
if (err) {
throw err;
}
console.log('Connected to database');
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
```
这将创建一个Express应用程序,并使用MySQL模块连接到数据库。
4. 添加路由
在app.js文件中添加路由:
```
// Get all books
app.get('/books', (req, res) => {
const sql = 'SELECT * FROM books';
db.query(sql, (err, result) => {
if (err) throw err;
res.send(result);
});
});
// Add a book
app.post('/books', (req, res) => {
const { title, author, year } = req.body;
const sql = `INSERT INTO books (title, author, year) VALUES ('${title}', '${author}', ${year})`;
db.query(sql, (err, result) => {
if (err) throw err;
res.send('Book added');
});
});
// Update a book
app.put('/books/:id', (req, res) => {
const { title, author, year } = req.body;
const sql = `UPDATE books SET title='${title}', author='${author}', year=${year} WHERE id=${req.params.id}`;
db.query(sql, (err, result) => {
if (err) throw err;
res.send('Book updated');
});
});
// Delete a book
app.delete('/books/:id', (req, res) => {
const sql = `DELETE FROM books WHERE id=${req.params.id}`;
db.query(sql, (err, result) => {
if (err) throw err;
res.send('Book deleted');
});
});
```
这些路由将允许您执行以下操作:
- 获取所有图书
- 添加一本书
- 更新一本书的信息
- 删除一本书
5. 测试应用程序
现在,您可以在终端中运行以下命令来启动应用程序:
```
node app.js
```
然后,您可以使用Postman或类似的工具测试应用程序中定义的路由。例如,您可以使用POST请求添加一本书:
```
POST http://localhost:3000/books
{
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald",
"year": 1925
}
```
然后,您可以使用GET请求获取所有图书:
```
GET http://localhost:3000/books
```
这将返回所有添加到books表的书籍信息。
希望这能帮助您开始创建自己的图书管理系统!
阅读全文
相关推荐
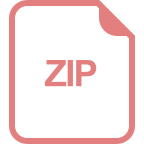
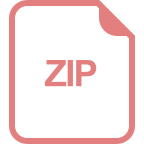
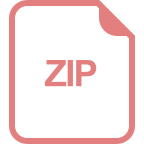
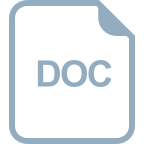







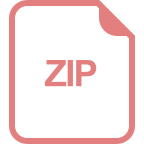
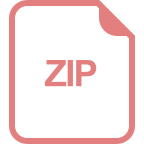
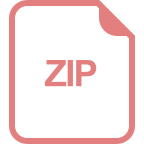
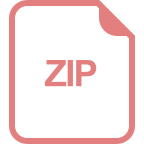
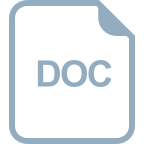