vue3 props传值 每次父组件的值变化子组件就会相应发送变化吗
时间: 2023-10-31 17:40:52 浏览: 149
在 Vue3 中,使用 `props` 传递数据时,如果父组件的值发生变化,子组件会相应地更新它接收到的值。但是,这并不意味着每次父组件的值变化就会立即发送变化,而是会在下一次组件更新周期时才进行更新。这是因为 Vue3 引入了响应式系统的优化,通过批量更新减少了不必要的更新操作,提高了性能。如果需要立即响应父组件值的变化,可以使用 `watch` 监听父组件的值变化并在回调函数中进行相应的操作。
相关问题
vue组件props传值子传父
在Vue中,父组件向子组件传递数据是通过props来实现的。而子组件向父组件传递数据,则需要通过自定义事件来实现。
首先,在父组件中,通过props属性将数据传递给子组件。可以在子组件标签上使用v-bind指令来动态绑定父组件的数据,或者直接在子组件标签上写入固定的值。
例如,在父组件中定义一个名为message的prop,并将一个字符串传递给子组件:
```vue
<template>
<div>
<child-component :message="parentMessage"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Hello from parent'
};
}
};
</script>
```
然后,在子组件中,可以通过props属性接收父组件传递过来的数据,并在模板中使用该数据。
```vue
<template>
<div>
<p>{{ message }}</p>
<button @click="sendMessageToParent">Send Message to Parent</button>
</div>
</template>
<script>
export default {
props: ['message'],
methods: {
sendMessageToParent() {
this.$emit('custom-event', 'Message from child');
}
}
};
</script>
```
在子组件中,可以使用`props`属性声明接收的属性名,然后在模板中直接使用即可。另外,如果需要向父组件发送数据,可以使用`$emit`方法触发一个自定义事件,并传递需要发送的数据。
最后,在父组件中,可以通过在子组件标签上监听自定义事件来接收子组件传递的数据。
```vue
<template>
<div>
<child-component :message="parentMessage" @custom-event="receiveMessageFromChild"></child-component>
<p>Message received from child: {{ childMessage }}</p>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Hello from parent',
childMessage: ''
};
},
methods: {
receiveMessageFromChild(message) {
this.childMessage = message;
}
}
};
</script>
```
这样,就完成了子组件向父组件传递数据的过程。当子组件中的按钮被点击时,将会触发`custom-event`事件,并将消息发送给父组件,父组件接收到消息后,将其保存在`childMessage`变量中,并在模板中进行展示。
vue子组件传值给父组件
Vue子组件向父组件传递数据的通信方式有很多,其中常用的有以下三种方法:
1. 使用props实现:父组件向子组件通过props传递一个函数,子组件触发事件时,调用这个函数,把自己的数据传给父组件,实质是进行了父子之间的相互通信。
2. 使用$emit实现:子组件通过$emit触发一个自定义事件,父组件在模板中使用v-on监听这个事件,并在对应的方法中获取子组件传递的数据。
3. 使用ref实现:给子组件的实例对象绑定一个自定义事件,子组件在触发事件时,调用这个函数,把自己的数据传给父组件。
阅读全文
相关推荐
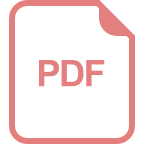













