vue中点击某一行,跳转到对应页面,并把当前行的id存入vuex中,在对应页面中获取到该id的值,举例说明
时间: 2023-08-31 17:44:02 浏览: 47
### 回答1:
假设我们有一个用户列表页面,每个用户都有一个对应的 id 值。我们希望用户点击某一行后跳转到用户详情页面,并且在用户详情页面中可以获取到当前用户的 id 值。
首先,在用户列表页面中,我们需要监听每一行的点击事件,并且将当前行的 id 值存入 Vuex 中。可以在每一行的模板中添加一个点击事件,如下所示:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr v-for="user in users" :key="user.id" @click="selectUser(user.id)">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
users: [
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' },
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
],
};
},
methods: {
selectUser(id) {
this.$store.commit('selectUser', id);
this.$router.push('/user/' + id);
},
},
};
</script>
```
在上面的代码中,我们使用了 `@click` 监听了每一行的点击事件,并且调用了 `selectUser` 方法。该方法首先将当前行的 id 值存入 Vuex 中(假设我们已经在 Vuex 中定义了一个 `selectedUserId` 的 state),然后使用 `$router.push` 跳转到用户详情页面,其中包含了当前用户的 id 值。
接下来,在用户详情页面中,我们可以使用 `$route.params` 获取到当前路由中的参数,也就是当前用户的 id 值。代码如下所示:
```html
<template>
<div>
<h1>User Details</h1>
<p>ID: {{ userId }}</p>
<!-- 其他用户详情信息 -->
</div>
</template>
<script>
export default {
computed: {
userId() {
return this.$route.params.id;
},
},
};
</script>
```
在上面的代码中,我们使用了一个计算属性 `userId`,该属性返回当前路由中的 `id` 参数。这样,在用户详情页面中,我们就可以获取到当前用户的 id 值了。
### 回答2:
在Vue中,实现点击某一行跳转到对应页面,并将当前行的id存入Vuex中,然后在对应页面中获取该id的值,可以通过以下步骤实现:
1. 首先,在数据源中准备好需要展示的数据,并通过v-for指令渲染到页面中的表格中,每一行添加点击事件,触发跳转和存储id的操作。
```vue
<template>
<div>
<table>
<tr v-for="item in dataList" @click="goToDetail(item.id)">
<td>{{item.name}}</td>
<td>{{item.age}}</td>
</tr>
</table>
</div>
</template>
<script>
export default {
data() {
return {
dataList: [
{ id: 1, name: '张三', age: 20 },
{ id: 2, name: '李四', age: 22 },
{ id: 3, name: '王五', age: 24 }
]
}
},
methods: {
goToDetail(id) {
this.$store.commit('saveId', id) // 将id存入Vuex
this.$router.push({ path: '/detail' }) // 跳转到对应页面
}
}
}
</script>
```
2. 在Vuex中创建一个module,用来存储和获取id的值。
```javascript
// store/modules/detail.js
const state = {
id: null
}
const mutations = {
saveId(state, id) {
state.id = id
}
}
export default {
namespaced: true,
state,
mutations
}
```
3. 在需要获取id的页面中,通过computed属性获取Vuex中存储的id值。
```vue
<template>
<div>
<p>当前ID:{{id}}</p>
</div>
</template>
<script>
export default {
computed: {
id() {
return this.$store.state.detail.id // 访问Vuex中存储的id值
}
}
}
</script>
```
这样,点击表格中的某一行后,会跳转到对应的页面,并将当前行的id存入Vuex中。在对应的页面中,可以通过computed属性获取到存储的id值。
相关推荐
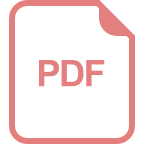
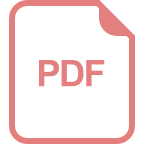














