pandas columns
时间: 2023-09-06 19:08:08 浏览: 71
In Pandas, columns refer to the variables or attributes that are present in a dataset. Each column represents a feature of the dataset and contains a set of values that correspond to that feature for every row in the dataset.
To access columns in Pandas, you can use the following syntax:
```python
import pandas as pd
# create a dataframe
df = pd.DataFrame({'Name': ['John', 'Mary', 'Peter'],
'Age': [28, 35, 42],
'Salary': [50000, 75000, 60000]})
# access a single column using square brackets and column name
name_col = df['Name']
# access multiple columns using a list of column names
age_salary_cols = df[['Age', 'Salary']]
```
In the above example, the `df` DataFrame has three columns: `Name`, `Age`, and `Salary`. To access a single column, you can use square brackets and the column name (e.g., `df['Name']`). To access multiple columns, you can pass a list of column names to the DataFrame (e.g., `df[['Age', 'Salary']]`).
You can also add new columns to a DataFrame using various operations, such as arithmetic operations, boolean operations, or by applying a function to an existing column.
```python
# add a new column using an arithmetic operation on existing columns
df['Bonus'] = df['Salary'] * 0.1
# add a new column using a boolean operation on existing columns
df['Seniority'] = df['Age'] >= 35
# add a new column by applying a function to an existing column
df['Name_Length'] = df['Name'].apply(len)
```
In the above example, we added three new columns to the `df` DataFrame. The `Bonus` column is created by multiplying the `Salary` column by 0.1. The `Seniority` column is created by checking if the `Age` column is greater than or equal to 35. The `Name_Length` column is created by applying the `len` function to each element in the `Name` column.
阅读全文
相关推荐
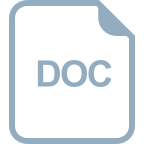
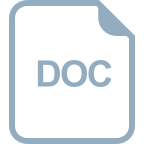
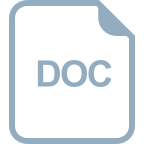








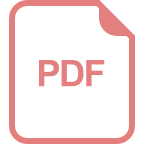
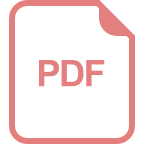
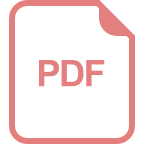




