安卓service如何能后台保活,请代码解释
时间: 2024-03-08 09:46:42 浏览: 151
在 Android 中,Service 是一种后台运行的组件,可以在后台持续运行。为了让 Service 能够在后台持续运行并保活,您可以使用以下方法:
1. 使用前台 Service:前台 Service 可以在系统状态栏显示一个通知,让用户知道该 Service 正在运行,从而避免被系统杀死。您可以在 Service 中使用 startForeground() 方法将其设置为前台 Service。
```
public class MyService extends Service {
private static final int NOTIFICATION_ID = 1;
@Override
public void onCreate() {
super.onCreate();
Notification notification = new Notification.Builder(this)
.setContentTitle("My Service")
.setContentText("Service is running")
.setSmallIcon(R.mipmap.ic_launcher)
.build();
startForeground(NOTIFICATION_ID, notification);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// 执行需要在后台持续运行的任务
return START_STICKY;
}
@Override
public void onDestroy() {
super.onDestroy();
stopForeground(true);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
2. 使用 AlarmManager:AlarmManager 是 Android 系统提供的一种调度服务,可以帮助您在特定的时间间隔内执行任务。您可以在 Service 中使用 AlarmManager,定时启动自己,从而避免被系统杀死。
```
public class MyService extends Service {
private static final long INTERVAL = 60 * 1000; // 每隔 1 分钟启动一次 Service
private static final int REQUEST_CODE = 1;
private static final String ACTION = "com.example.myapp.MyService";
private AlarmManager alarmManager;
private PendingIntent pendingIntent;
@Override
public void onCreate() {
super.onCreate();
alarmManager = (AlarmManager) getSystemService(ALARM_SERVICE);
Intent intent = new Intent(this, MyService.class);
intent.setAction(ACTION);
pendingIntent = PendingIntent.getService(this, REQUEST_CODE, intent, 0);
alarmManager.setRepeating(AlarmManager.RTC_WAKEUP, System.currentTimeMillis(), INTERVAL, pendingIntent);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// 执行需要在后台持续运行的任务
return START_STICKY;
}
@Override
public void onDestroy() {
super.onDestroy();
alarmManager.cancel(pendingIntent);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
需要注意的是,使用以上方法运行的 Service 都需要在 AndroidManifest.xml 文件中注册,并且应用程序需要在启动时启动 Service。另外,如果您需要在后台执行网络请求等耗时操作,建议使用 IntentService 或 AsyncTask 等异步方式进行处理,以免阻塞主线程。
阅读全文
相关推荐
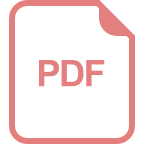

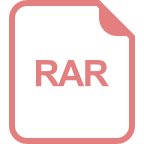
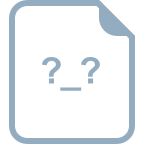
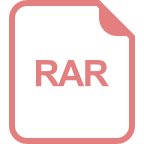
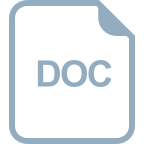




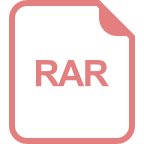
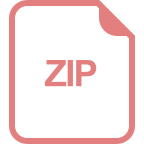
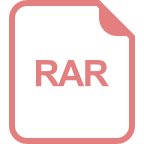



