用.net core webapi 实现 数据的增删改查 的案例
时间: 2024-03-17 21:45:25 浏览: 15
好的,我可以为您提供一个简单的用.NET Core WebAPI 实现数据的增删改查的案例。
首先,我们需要创建一个.NET Core WebAPI 项目。在Visual Studio中,选择“新建项目”->“.NET Core”->“ASP.NET Core Web Application”。然后,选择“API”模板并命名项目。
接下来,我们需要创建一个数据模型。在这个案例中,我们将使用一个简单的“TodoItem”模型,其中包含一个“Id”和一个“Name”属性。我们在Models文件夹中创建一个名为“TodoItem.cs”的类,并添加以下代码:
```csharp
public class TodoItem
{
public int Id { get; set; }
public string Name { get; set; }
}
```
然后,我们需要创建一个控制器来处理数据。在Controllers文件夹中创建一个名为“TodoItemsController.cs”的类,并添加以下代码:
```csharp
[Route("api/[controller]")]
[ApiController]
public class TodoItemsController : ControllerBase
{
private readonly List<TodoItem> _items = new List<TodoItem>();
[HttpGet]
public ActionResult<IEnumerable<TodoItem>> Get()
{
return _items;
}
[HttpGet("{id}")]
public ActionResult<TodoItem> GetById(int id)
{
var item = _items.FirstOrDefault(i => i.Id == id);
if (item == null)
{
return NotFound();
}
return item;
}
[HttpPost]
public ActionResult<TodoItem> Post(TodoItem item)
{
item.Id = _items.Count + 1;
_items.Add(item);
return CreatedAtAction(nameof(GetById), new { id = item.Id }, item);
}
[HttpPut("{id}")]
public ActionResult<TodoItem> Put(int id, TodoItem item)
{
var existingItem = _items.FirstOrDefault(i => i.Id == id);
if (existingItem == null)
{
return NotFound();
}
existingItem.Name = item.Name;
return NoContent();
}
[HttpDelete("{id}")]
public ActionResult<TodoItem> Delete(int id)
{
var existingItem = _items.FirstOrDefault(i => i.Id == id);
if (existingItem == null)
{
return NotFound();
}
_items.Remove(existingItem);
return existingItem;
}
}
```
这个控制器包含了HTTP GET、POST、PUT和DELETE方法。它使用一个简单的List<TodoItem>来存储数据,并且每个方法都执行相应的操作。
最后,我们需要在Startup.cs文件中配置路由。在Configure方法中添加以下代码:
```csharp
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
```
现在,我们可以启动应用程序并使用Postman或其他HTTP客户端测试API。例如,我们可以使用以下请求创建一个新的TodoItem:
```
POST https://localhost:5001/api/todoitems
Content-Type: application/json
{
"name": "Buy milk"
}
```
然后,我们可以使用以下请求获取所有TodoItems:
```
GET https://localhost:5001/api/todoitems
```
这就是一个简单的.NET Core WebAPI实现数据的增删改查的案例。
相关推荐
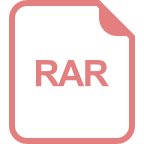
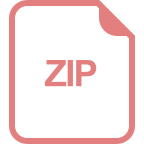














