python字符型五子棋人工对战
时间: 2023-10-08 16:11:14 浏览: 61
好的,我可以帮你实现这个功能。你需要安装 Python 3.x 和 Pygame 库。下面是一个简单的 Python 代码示例,用于实现字符型五子棋人工对战:
```python
import pygame
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子大小
STONE_SIZE = 30
# 定义线条颜色
LINE_COLOR = (0, 0, 0)
# 定义棋子颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 初始化 Pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((BOARD_SIZE * STONE_SIZE, BOARD_SIZE * STONE_SIZE))
# 设置窗口标题
pygame.display.set_caption('五子棋')
# 定义棋盘
board = [[0] * BOARD_SIZE for i in range(BOARD_SIZE)]
# 定义当前玩家,1 表示黑子,-1 表示白子
current_player = 1
# 画棋盘
def draw_board():
screen.fill(WHITE)
for i in range(BOARD_SIZE):
pygame.draw.line(screen, LINE_COLOR, (STONE_SIZE, (i + 1) * STONE_SIZE), ((BOARD_SIZE - 1) * STONE_SIZE, (i + 1) * STONE_SIZE))
pygame.draw.line(screen, LINE_COLOR, ((i + 1) * STONE_SIZE, STONE_SIZE), ((i + 1) * STONE_SIZE, (BOARD_SIZE - 1) * STONE_SIZE))
# 画棋子
def draw_stone(x, y):
if board[x][y] == 1:
pygame.draw.circle(screen, BLACK, ((x + 1) * STONE_SIZE, (y + 1) * STONE_SIZE), STONE_SIZE // 2)
elif board[x][y] == -1:
pygame.draw.circle(screen, WHITE, ((x + 1) * STONE_SIZE, (y + 1) * STONE_SIZE), STONE_SIZE // 2)
# 判断是否胜利
def is_win(x, y):
# 判断行
count = 0
for i in range(BOARD_SIZE):
if board[i][y] == board[x][y]:
count += 1
else:
count = 0
if count == 5:
return True
# 判断列
count = 0
for j in range(BOARD_SIZE):
if board[x][j] == board[x][y]:
count += 1
else:
count = 0
if count == 5:
return True
# 判断左上到右下的斜线
count = 0
for i in range(BOARD_SIZE):
if x + i >= BOARD_SIZE or y + i >= BOARD_SIZE:
break
if board[x + i][y + i] == board[x][y]:
count += 1
else:
count = 0
if count == 5:
return True
# 判断右上到左下的斜线
count = 0
for i in range(BOARD_SIZE):
if x - i < 0 or y + i >= BOARD_SIZE:
break
if board[x - i][y + i] == board[x][y]:
count += 1
else:
count = 0
if count == 5:
return True
return False
# 主循环
while True:
for event in pygame.event.get():
# 退出程序
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 鼠标点击事件
if event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
x //= STONE_SIZE
y //= STONE_SIZE
if board[x][y] == 0:
board[x][y] = current_player
draw_stone(x, y)
if is_win(x, y):
print('黑方胜利' if current_player == 1 else '白方胜利')
pygame.quit()
exit()
current_player = -current_player
draw_board()
pygame.display.update()
```
运行代码后,你可以看到一个字符型的五子棋游戏窗口。你可以使用鼠标点击空白区域来下棋,游戏会自动判断胜负并结束游戏。
阅读全文
相关推荐

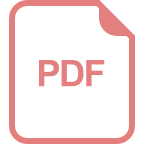
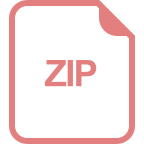
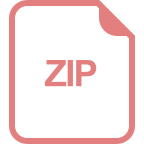
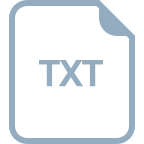
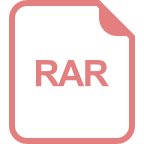
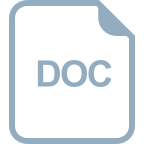
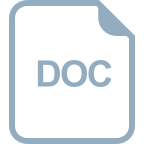
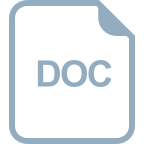
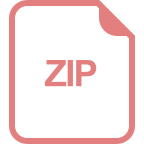
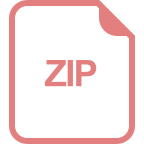
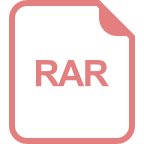
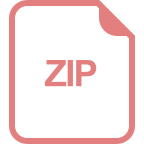
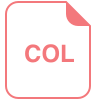
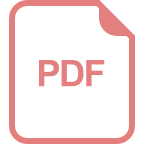