用python写一个模拟砍价
时间: 2023-06-02 12:05:29 浏览: 377
好的,我可以帮助你回答这个问题。首先,模拟砍价需要建立一个价格模型,可以使用Python中的随机数生成器来模拟商品的价值。然后,可以建立一个砍价函数,在函数中使用随机数生成器产生一个砍价的价格,然后和当前的价格进行比较,判断砍价是否成功。最后,可以使用一个循环来模拟反复砍价直到达成协议或砍价失败。如果您需要更具体的帮助,请告诉我您需要的详细信息。
相关问题
用python写一段模拟砍价的代码
好的,以下是一段简单的模拟砍价的 Python 代码:
```python
import random
price = 100 # 商品售价
while True:
offer = int(input("请输入您的出价:"))
if offer > price:
print("出价高于商品售价,请重新出价!")
elif offer == price:
print("恭喜您!砍价成功,您成功购买此商品!")
break
else:
print("出价低于商品售价!")
if random.random() > 0.5:
price = price - random.randint(1, 10)
print("商家已接受您的出价,但是只能给您减少 %d 元,商品价格为 %d 元。" % (price - offer, price))
else:
print("非常抱歉,商家拒绝了您的出价!")
```
这段代码模拟了用户输入出价,商家接受或拒绝出价的过程。如果商家接受出价,则随机给出一个减价的幅度,商品的售价会相应减少;否则,用户需要重新出价。
python模拟砍价
在Python中模拟砍价游戏通常会涉及到一些基本的游戏规则和随机元素。你可以设计一个简单的交互式程序,让用户扮演买家和卖家的角色,通过输入价格和砍价策略来进行互动。以下是一个简化的步骤:
1. **创建角色**:定义两个类,如Buyer和Seller,每个类包含初始价格、折扣策略等属性。
```python
class Buyer:
def __init__(self, initial_price):
self.price = initial_price
# 设定砍价次数或折扣范围
class Seller:
def __init__(self, initial_price):
self.price = initial_price
# 设定最低接受价格或折扣范围
```
2. **用户输入**:设置循环让玩家轮流砍价。买家猜测价格,卖家给出回应。
```python
def simulate_bargaining():
buyer = Buyer(random.randint(50, 100)) # 示例:随机价格在50到100之间
seller = Seller(buyer.price)
while True:
print(f"买家认为价格应该是{buyer.price}")
new_price = input("请输入您的出价(输入'quit'退出):")
if new_price.lower() == "quit":
break
if not new_price.isdigit():
print("无效输入,请输入数字")
continue
new_price = int(new_price)
buyer.price = max(new_price, seller.accepted_min_price) # 确保买家不会低于卖家底线
seller.price -= random.randint(1, max(seller.discount_range, buyer.price - seller.price)) # 卖家降价
```
3. **结束条件**:当买家不再砍价或者达到某个预设条件(比如达到协议价格),游戏结束。
4. **结果展示**:显示最终交易价格,并询问玩家是否满意。
这只是一个基础版本,实际模拟可以更复杂,比如添加更多谈判策略、历史记录跟踪等。
阅读全文
相关推荐
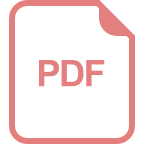
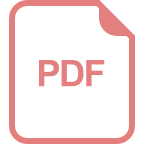






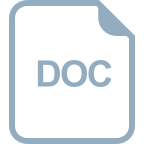
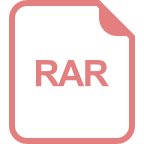
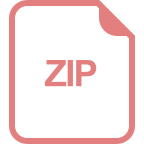
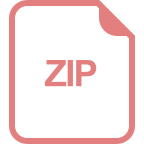
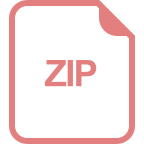
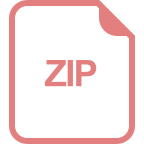
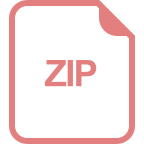
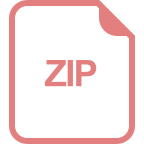