用py 写一个植物大战僵尸的游戏
时间: 2023-12-07 13:06:08 浏览: 69
好的,植物大战僵尸是一款非常经典的游戏,我可以给你提供一些关键的代码实现,但是由于篇幅限制,无法给你提供完整的代码,希望你可以通过以下代码实现自己的植物大战僵尸游戏。
首先,我们需要导入一些必要的模块:
``` python
import pygame
import sys
import random
```
接着,我们需要初始化 pygame:
``` python
pygame.init()
```
然后,我们需要定义一些常量:
``` python
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
FPS = 60
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
```
接着,我们需要创建游戏窗口:
``` python
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("植物大战僵尸")
```
然后,我们需要定义一些类:
``` python
class Plant(pygame.sprite.Sprite):
def __init__(self, x, y, image):
pygame.sprite.Sprite.__init__(self)
self.image = image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
pass
class Zombie(pygame.sprite.Sprite):
def __init__(self, x, y, image):
pygame.sprite.Sprite.__init__(self)
self.image = image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
def update(self):
pass
```
最后,我们需要编写游戏主循环:
``` python
def main():
clock = pygame.time.Clock()
# 创建精灵组
all_sprites = pygame.sprite.Group()
# 创建植物
plant_image = pygame.image.load("plant.png").convert_alpha()
plant = Plant(100, 100, plant_image)
all_sprites.add(plant)
# 创建僵尸
zombie_image = pygame.image.load("zombie.png").convert_alpha()
zombie = Zombie(700, 100, zombie_image)
all_sprites.add(zombie)
running = True
while running:
clock.tick(FPS)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新精灵组
all_sprites.update()
# 绘制背景
screen.fill(WHITE)
# 绘制精灵组
all_sprites.draw(screen)
# 更新屏幕
pygame.display.flip()
pygame.quit()
sys.exit()
if __name__ == "__main__":
main()
```
这样,我们就完成了一个简单的植物大战僵尸游戏的代码实现。当然,这只是一个非常简单的实现,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
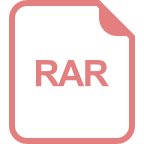



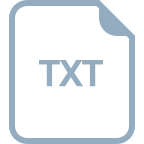
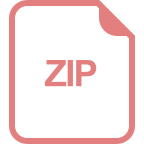
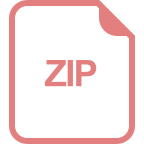
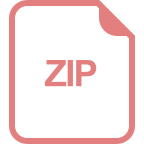
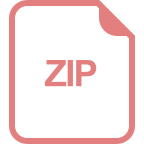
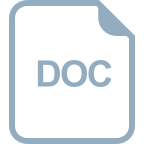
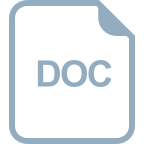
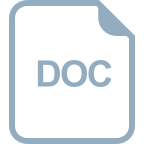
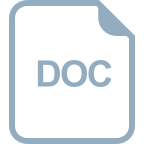
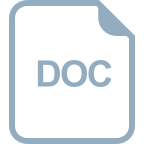

