有数据库工具类,jsp页面获取数据库里的字段并以表格形式显示,完整代码怎么写
时间: 2024-02-29 20:53:31 浏览: 128
好的,下面是一个简单的示例代码,用于实现从数据库中获取数据并在 JSP 页面中以表格形式显示:
1. 数据库连接工具类(DBUtil.java):
```java
import java.sql.*;
public class DBUtil {
private static final String DRIVER_NAME = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/mydb";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
public static Connection getConnection() throws ClassNotFoundException, SQLException {
Class.forName(DRIVER_NAME);
return DriverManager.getConnection(DB_URL, USERNAME, PASSWORD);
}
public static void closeConnection(Connection conn, Statement stmt, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
2. JSP 页面(index.jsp):
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Database Table</title>
</head>
<body>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<%
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
conn = DBUtil.getConnection();
stmt = conn.createStatement();
String sql = "SELECT * FROM users";
rs = stmt.executeQuery(sql);
while (rs.next()) {
%>
<tr>
<td><%=rs.getInt("id")%></td>
<td><%=rs.getString("name")%></td>
<td><%=rs.getInt("age")%></td>
</tr>
<%
}
} catch (Exception e) {
e.printStackTrace();
} finally {
DBUtil.closeConnection(conn, stmt, rs);
}
%>
</tbody>
</table>
</body>
</html>
```
这个示例使用了 MySQL 数据库,并假设数据库名为 "mydb",表名为 "users",包含三个字段:ID、Name 和 Age。在 JSP 页面中,使用 JDBC 连接数据库,执行 SQL 查询语句,遍历结果集并以表格形式显示。需要注意的是,这个示例中只是简单地演示了如何从数据库中获取数据并在 JSP 页面中显示,实际项目中需要根据需要进行适当的修改和优化。
阅读全文
相关推荐
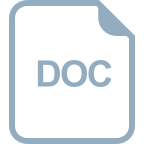
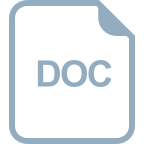
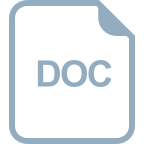
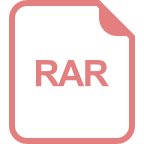
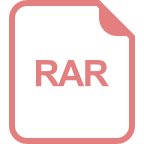
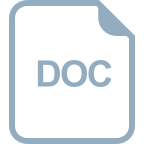
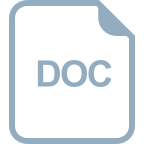
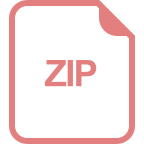
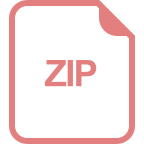
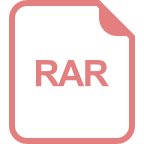
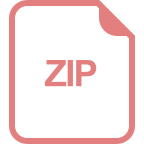
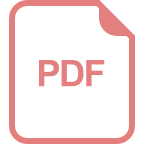
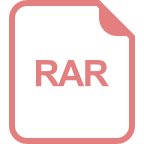
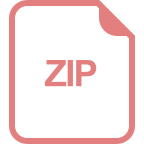
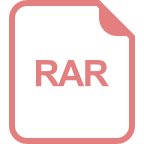
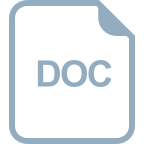
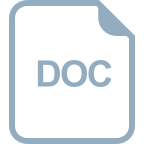
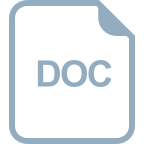
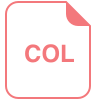