yield from torch.randperm(n, generator=generator).tolist() MemoryError
时间: 2024-05-22 21:10:43 浏览: 219
这个错误通常表示在尝试生成长度为n的随机排列时,内存不足。这可能是因为n太大,导致了随机排列的列表过大,无法被计算机内存所容纳。
为了解决这个问题,你可以考虑以下几种方法:
1. 减小n的值,或者使用更小的数据集来测试代码;
2. 优化代码,例如使用生成器而不是列表等;
3. 增加计算机内存,例如使用更高配置的计算机或者云服务。
如果仍然无法解决问题,建议你查看其他相关错误提示或者调用堆栈信息,以便更好地定位问题所在。
相关问题
Write a generator that outputs the hailstone sequence from hw01. Here's a quick reminder of how the hailstone sequence is defined: Pick a positive integer n as the start. If n is even, divide it by 2. If n is odd, multiply it by 3 and add 1. Continue this process until n is 1. For some extra practice, try writing a solution using recursion. Since hailstone returns a generator, you can yield from a call to hailstone!
Sure! Here's an implementation of the `hailstone` generator function:
```python
def hailstone(n):
yield n
while n != 1:
n = n // 2 if n % 2 == 0 else 3 * n + 1
yield n
```
This generator function takes a positive integer `n` as the start of the hailstone sequence, and generates each subsequent number in the sequence until it reaches 1.
The function first yields the starting number `n`, and then enters a loop that continues until `n` is equal to 1. In each iteration of the loop, the function computes the next number in the sequence based on whether `n` is even or odd, and yields that number. If `n` is even, the function divides it by 2 using integer division (`//`). If `n` is odd, the function multiplies it by 3 and adds 1.
Here's an example usage of this generator function:
```python
>>> list(hailstone(10))
[10, 5, 16, 8, 4, 2, 1]
```
This example generates the hailstone sequence starting from 10, which is `[10, 5, 16, 8, 4, 2, 1]`. The `list` function is used to consume all the elements of the generator and return them as a list.
阅读全文
相关推荐
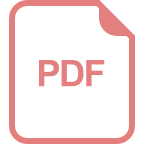
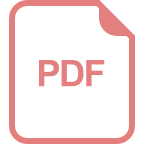
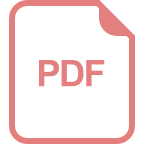
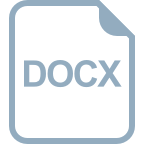
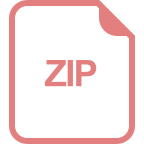
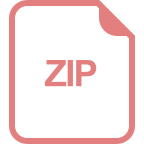
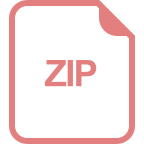
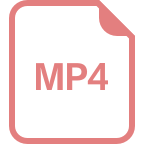
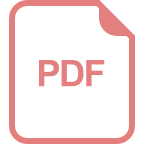
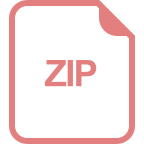
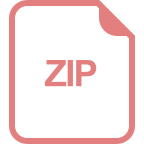