STM32F103VCT编写程序,在串口助手中输入电压值,OLED屏幕上显示相应电压
时间: 2024-03-14 14:45:35 浏览: 172
这里提供一个简单的程序框架,可以根据具体需要进行修改。
首先,需要初始化串口和OLED屏幕,以及ADC模块。假设使用的是USART1,OLED屏幕使用的是I2C接口,ADC模块使用的是PA0通道:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "oled.h"
void USART1_Init(void);
void ADC1_Init(void);
int main(void)
{
float voltage = 0.0;
char buffer[32] = {0};
USART1_Init();
OLED_Init();
ADC1_Init();
while(1)
{
// 读取ADC采样值并计算电压值
uint16_t adc_value = ADC_GetConversionValue(ADC1);
voltage = adc_value * 3.3 / 4096.0;
// 将电压值转换为字符串并发送到串口
sprintf(buffer, "Voltage: %.2fV\r\n", voltage);
USART_SendString(USART1, buffer);
// 在OLED屏幕上显示电压值
OLED_Clear();
OLED_ShowString(0, 0, "Voltage:");
OLED_ShowFloat(64, 0, voltage, 2);
OLED_ShowString(96, 0, "V");
OLED_Refresh();
// 延时一段时间
Delay_ms(500);
}
}
void USART1_Init(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void ADC1_Init(void)
{
ADC_InitTypeDef ADC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
ADC_DeInit(ADC1);
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfChannel = 1;
ADC_Init(ADC1, &ADC_InitStructure);
ADC_Cmd(ADC1, ENABLE);
ADC_ResetCalibration(ADC1);
while(ADC_GetResetCalibrationStatus(ADC1));
ADC_StartCalibration(ADC1);
while(ADC_GetCalibrationStatus(ADC1));
}
void Delay_ms(uint32_t ms)
{
uint32_t i, j;
for(i = 0; i < ms; i++)
for(j = 0; j < 7200; j++);
}
```
在上面的程序中,使用了一个自定义函数USART_SendString()用于向串口发送字符串,以及一个自定义函数OLED_ShowFloat()用于在OLED屏幕上显示浮点数。这两个函数的具体实现可以根据实际情况进行修改。
此外,程序中还使用了一个简单的延时函数Delay_ms()来控制程序的运行速度。如果需要更精确的延时,可以使用定时器或者操作系统提供的延时函数。
阅读全文
相关推荐
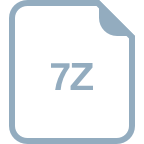


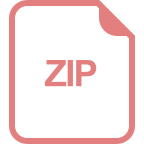
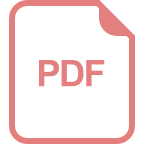
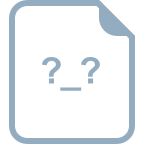
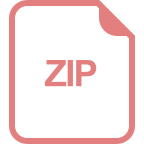
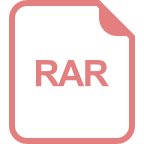
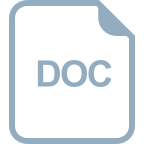


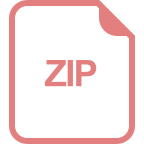
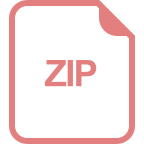
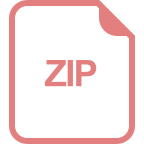
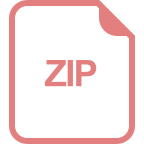