如何使用java springboot 整合mybatis/mybatis-plus
时间: 2023-11-09 09:17:33 浏览: 41
你可以按照以下步骤来使用Java Spring Boot整合MyBatis/MyBatis-Plus:
1. 添加MyBatis/MyBatis-Plus依赖
在pom.xml文件中添加以下依赖:
```xml
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- MyBatis-Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.0</version>
</dependency>
```
2. 配置数据源
在application.properties或application.yml文件中配置数据源信息,例如:
```yaml
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/mybatis_plus?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=GMT%2B8
username: root
password: root
```
3. 配置MyBatis/MyBatis-Plus
在配置类中添加以下注解:
```java
@Configuration
@MapperScan("com.example.demo.mapper")
public class MybatisPlusConfig {
}
```
其中com.example.demo.mapper为你的Mapper包路径。
4. 编写Mapper接口和XML文件
编写Mapper接口和XML文件,例如:
```java
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.demo.entity.User">
<id column="id" property="id" />
<result column="name" property="name" />
<result column="age" property="age" />
<result column="email" property="email" />
</resultMap>
<select id="selectById" resultMap="BaseResultMap">
select * from user where id = #{id}
</select>
<select id="selectList" resultMap="BaseResultMap">
select * from user
</select>
<insert id="insert" parameterType="com.example.demo.entity.User">
insert into user (name, age, email) values (#{name}, #{age}, #{email})
</insert>
<update id="updateById" parameterType="com.example.demo.entity.User">
update user set name = #{name}, age = #{age}, email = #{email} where id = #{id}
</update>
<delete id="deleteById" parameterType="java.lang.Long">
delete from user where id = #{id}
</delete>
</mapper>
```
5. 编写Service和Controller
编写Service和Controller,例如:
```java
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
}
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getById(id);
}
@GetMapping("/")
public List<User> getUserList() {
return userService.list();
}
@PostMapping("/")
public boolean addUser(@RequestBody User user) {
return userService.save(user);
}
@PutMapping("/")
public boolean updateUser(@RequestBody User user) {
return userService.updateById(user);
}
@DeleteMapping("/{id}")
public boolean deleteUserById(@PathVariable Long id) {
return userService.removeById(id);
}
}
```
6. 运行程序
运行程序,访问http://localhost:8080/user/即可进行测试。
以上就是使用Java Spring Boot整合MyBatis/MyBatis-Plus的步骤,希望对你有帮助。
相关推荐
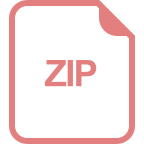
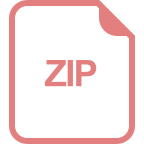
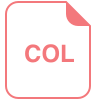
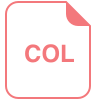
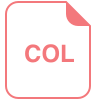
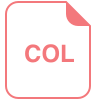
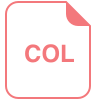









