qt 按钮背景颜色变化
时间: 2023-07-05 18:31:41 浏览: 521
在Qt中,可以使用样式表来设置按钮的背景颜色,从而实现按钮按下时背景颜色的变化。具体步骤如下:
1. 在Qt的界面设计器中,选中要设置背景颜色的QPushButton控件;
2. 在属性面板中,找到“styleSheet”属性,点击旁边的“编辑”按钮;
3. 在弹出的样式表编辑器中,输入以下代码(以设置按钮按下后变成灰色为例):
```
QPushButton:pressed {
background-color: gray;
}
```
4. 点击“确定”按钮,保存样式表设置。
这样,在运行程序后,当用户点击这个按钮时,就会看到按钮的背景颜色变成了灰色,表示按钮已经被按下了。如果需要设置按钮释放后的背景颜色,可以使用类似以下代码:
```
QPushButton {
background-color: blue;
}
QPushButton:pressed {
background-color: gray;
}
```
这样,在按钮按下时背景颜色变成灰色,在按钮释放时背景颜色又变成了蓝色。
相关问题
qt按钮点击改变颜色
在Qt中,你可以轻松地为按钮添加单击事件处理程序,使得按钮被点击后颜色发生变化。以下是步骤和基本示例:
1. **包含所需库**:
在`.pro`文件或`.cpp`文件中,确保包含了所需的`QApplication`、`QPushButton`和可能的`QtWidgets`库。
2. **创建按钮并设置样式**:
创建一个QPushButton实例,并为其定义初始的颜色。例如:
```cpp
QPushButton* button = new QPushButton("点击我", this);
button->setStyleSheet("background-color: blue;");
```
这里将按钮背景设为蓝色。
3. **添加点击事件处理函数**:
使用`QObject::connect()`方法连接按钮的`clicked()`信号到一个槽函数(slot function),该槽函数会更改按钮的颜色:
```cpp
connect(button, &QPushButton::clicked, [button](){
// 改变颜色的部分
QColor initialColor = button->palette().color(QPalette::Button); // 获取当前颜色
QColor newColor = initialColor.darker(); // 或者用其他方式改变颜色,比如更亮或不同颜色
button->setStyleSheet("background-color: " + newColor.name()); // 更新样式
});
```
当按钮被点击时,这个槽函数会被调用,使按钮颜色变化。
4. **完整示例**:
```cpp
#include <QApplication>
#include <QPushButton>
#include <QPalette>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QWidget window;
window.resize(200, 100);
QPushButton button("点击我", &window);
button.move(50, 50);
button.setStyleSheet("background-color: blue;");
QObject::connect(&button, &QPushButton::clicked, [button](){
QColor initialColor = button.palette().color(QPalette::Button);
QColor newColor = initialColor.darker();
button.setStyleSheet("background-color: " + newColor.name());
});
window.show();
return app.exec();
}
```
以上代码中,每次按钮被点击,按钮的颜色都会暗淡一些。你可以根据需要修改颜色变化的方式。
qtbutton颜色变化动画
在Qt中,要实现QPushButton的颜色变化动画,可以使用QPropertyAnimation和QObject的槽函数配合。首先,你需要设置一个信号连接到QPushButton的点击事件,然后在槽函数中修改按钮的背景色,并创建一个动画对象。
以下是一个简单的示例:
```cpp
#include <QApplication>
#include <QWidget>
#include <QPushButton>
#include <QPropertyAnimation>
class ButtonColorChange : public QWidget {
public:
explicit ButtonColorChange(QWidget *parent = nullptr)
: QWidget(parent), button(new QPushButton("Click me", this)) {
connect(button, &QPushButton::clicked, this, [this]() {
startColorAnimation();
});
}
private slots:
void startColorAnimation() {
// 设置初始颜色
QColor initialColor = button->palette().color(QPalette::Button);
// 创建动画
QPropertyAnimation* animation = new QPropertyAnimation(button, "palette");
animation->setDuration(500); // 动画持续时间,单位毫秒
animation->setStartValue(initialColor);
animation->setEndValue(QColor("#ff0000")); // 目标颜色,例如红色
// 开始动画
animation->start();
}
private:
QPushButton* button;
};
int main(int argc, char **argv) {
QApplication app(argc, argv);
ButtonColorChange window;
window.show();
return app.exec();
}
```
在这个例子中,当用户点击按钮时,会触发`startColorAnimation`槽函数,该函数中创建了一个QPropertyAnimation对象,设置了初始颜色、目标颜色以及动画时长。动画会在指定时间内逐渐改变QPushButton的背景颜色。
阅读全文
相关推荐
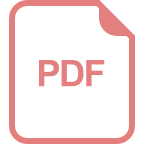
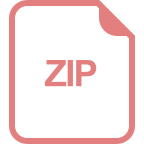
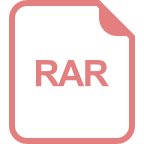
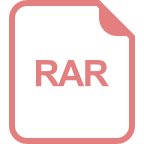
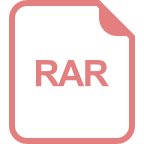
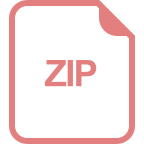
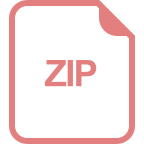
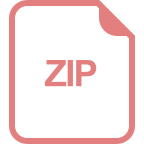
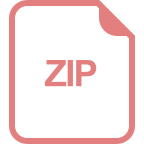
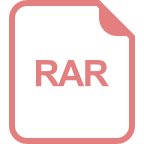
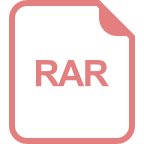
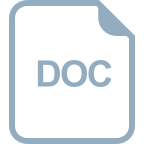
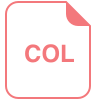

