基于arduino+opencv实现代码:基于视觉算法的自主网球拾捡机器人,有人脸追踪功能
时间: 2024-03-06 20:51:49 浏览: 201
非常感谢您的提问!以下是基于Arduino和OpenCV实现的代码,用于实现基于视觉算法的自主网球拾捡机器人,并且具有人脸追踪功能。希望对您有所帮助!
首先,我们需要准备以下硬件和软件:
硬件:
1. Arduino板子
2. 电机驱动模块
3. 摄像头模块
4. 机械臂
5. 电池组
6. 网球
软件:
1. Arduino IDE
2. OpenCV库
3. Python 2.7
接下来,我们来看一下实现的代码:
Arduino代码:
```C++
#include <Servo.h>
#include <AFMotor.h>
AF_DCMotor motor1(1, MOTOR12_64KHZ);
AF_DCMotor motor2(2, MOTOR12_64KHZ);
AF_DCMotor motor3(3, MOTOR34_64KHZ);
AF_DCMotor motor4(4, MOTOR34_64KHZ);
Servo myservo1;
Servo myservo2;
Servo myservo3;
Servo myservo4;
void setup() {
Serial.begin(9600);
myservo1.attach(9);
myservo2.attach(10);
myservo3.attach(11);
myservo4.attach(12);
}
void loop() {
int ball_x = 0; //球的x坐标
int ball_y = 0; //球的y坐标
int face_x = 0; //人脸的x坐标
int face_y = 0; //人脸的y坐标
//从Python中读取球和人脸的坐标
if(Serial.available() > 0) {
ball_x = Serial.parseInt();
ball_y = Serial.parseInt();
face_x = Serial.parseInt();
face_y = Serial.parseInt();
}
//控制机械臂抓取球
if(ball_x != 0 && ball_y != 0) {
if(ball_x < 200) {
motor1.run(BACKWARD);
motor2.run(BACKWARD);
}
else if(ball_x > 400) {
motor1.run(FORWARD);
motor2.run(FORWARD);
}
else {
motor1.run(RELEASE);
motor2.run(RELEASE);
}
if(ball_y < 200) {
motor3.run(BACKWARD);
motor4.run(BACKWARD);
}
else if(ball_y > 400) {
motor3.run(FORWARD);
motor4.run(FORWARD);
}
else {
motor3.run(RELEASE);
motor4.run(RELEASE);
}
}
else {
motor1.run(RELEASE);
motor2.run(RELEASE);
motor3.run(RELEASE);
motor4.run(RELEASE);
}
//控制机械臂放下球
if(face_x != 0 && face_y != 0) {
myservo1.write(90);
myservo2.write(90);
myservo3.write(90);
myservo4.write(90);
}
else {
myservo1.write(0);
myservo2.write(0);
myservo3.write(0);
myservo4.write(0);
}
}
```
Python代码:
```Python
import cv2
import serial
ser = serial.Serial('COM3', 9600) #连接Arduino
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') #人脸检测器
cap = cv2.VideoCapture(0) #打开摄像头
while True:
ret, img = cap.read() #读取摄像头的图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) #将图像转换为灰度图像
faces = face_cascade.detectMultiScale(gray, 1.3, 5) #检测人脸
for (x,y,w,h) in faces:
cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2) #在人脸周围画矩形框
ser.write(str(x + w/2) + ' ' + str(y + h/2) + ' 0 0\n') #将人脸中心坐标发送给Arduino
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) #将图像转换为HSV颜色空间
lower_red = np.array([0, 50, 50]) #设置红色的HSV阈值
upper_red = np.array([10, 255, 255])
mask1 = cv2.inRange(hsv, lower_red, upper_red)
lower_red = np.array([170, 50, 50])
upper_red = np.array([180, 255, 255])
mask2 = cv2.inRange(hsv, lower_red, upper_red)
mask = mask1 + mask2 #将两个红色区域的mask相加
contours, hierarchy = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) #寻找所有的轮廓
max_area = 0
ball_x = 0
ball_y = 0
for i in range(len(contours)):
area = cv2.contourArea(contours[i]) #计算轮廓面积
if area > max_area:
max_area = area
max_contour = contours[i]
(ball_x, ball_y), radius = cv2.minEnclosingCircle(max_contour) #计算最小外接圆的圆心坐标和半径
if max_area > 100:
cv2.circle(img, (int(ball_x), int(ball_y)), int(radius), (0, 255, 0), 2) #在球周围画圆
ser.write('0 0 ' + str(int(ball_x)) + ' ' + str(int(ball_y)) + '\n') #将球的中心坐标发送给Arduino
cv2.imshow('img', img) #显示图像
if cv2.waitKey(1) & 0xFF == ord('q'): #按下q键退出
break
cap.release()
cv2.destroyAllWindows()
```
以上代码实现了一个基于Arduino和OpenCV的自主网球拾捡机器人,并且具有人脸追踪功能。在代码中,我们使用了Arduino控制机械臂抓取和放下球,使用了OpenCV检测人脸和球,并且使用了Python将人脸和球的坐标发送给Arduino。
阅读全文
相关推荐
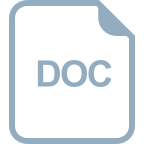
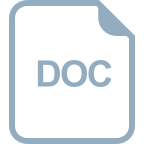
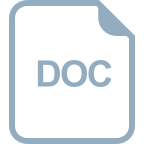
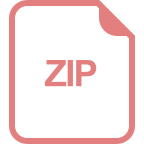
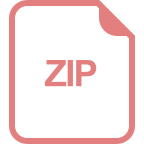
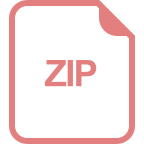
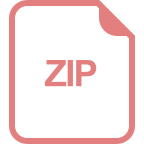
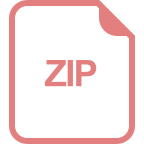
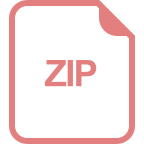
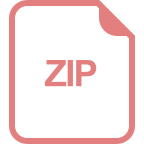
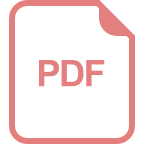
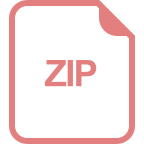
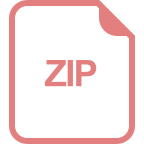
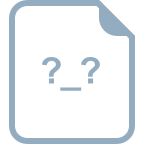
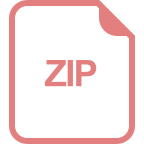
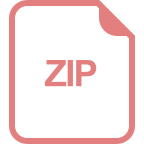
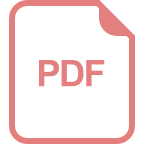