java 生成二维码qr_code.png,包含订单号R2022121200000027
时间: 2023-12-14 15:36:35 浏览: 27
要在 Java 中生成二维码,可以使用第三方库Zxing。首先需要在项目中引入该库,可以通过 Maven 或 Gradle 进行引入。
生成二维码的代码如下:
```java
import java.io.File;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.Map;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
public class QRCodeGenerator {
public static void main(String[] args) {
String qrCodeData = "R2022121200000027";
String filePath = "qr_code.png";
int size = 250;
String fileType = "png";
File qrFile = new File(filePath);
try {
createQRCode(qrFile, qrCodeData, size, fileType);
System.out.println("QR Code generated successfully!");
} catch (WriterException e) {
System.out.println("Error generating QR Code: " + e.getMessage());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
private static void createQRCode(File qrFile, String qrCodeData, int size, String fileType)
throws WriterException, Exception {
// Set QR code parameters
Map<EncodeHintType, Object> hintMap = new HashMap<EncodeHintType, Object>();
hintMap.put(EncodeHintType.CHARACTER_SET, "UTF-8");
hintMap.put(EncodeHintType.MARGIN, 2);
hintMap.put(EncodeHintType.QR_VERSION, 4);
// Create QR code writer and encode the data
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix byteMatrix = qrCodeWriter.encode(qrCodeData, BarcodeFormat.QR_CODE, size, size, hintMap);
// Create QR code image
Path path = Paths.get(qrFile.getAbsolutePath());
MatrixToImageWriter.writeToPath(byteMatrix, fileType, path);
}
}
```
在上面的代码中,我们首先定义了要生成的二维码的内容、文件路径、大小和文件类型。然后使用 QRCodeWriter 对象将内容编码为二维码,再使用 MatrixToImageWriter 将其写入文件中。运行该代码,会在项目根目录下生成一个名为 qr_code.png 的二维码文件,包含订单号 R2022121200000027。
你也可以根据自己的需求修改代码中的参数,比如大小、文件类型等。
相关推荐
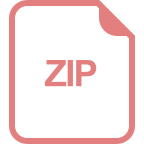
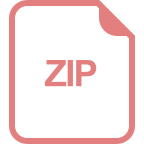
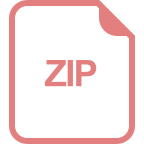














