请结合给出的素材,实现图9-59所示的“焦 点图轮播”效果,具体要求如下。 (1)当页面加载完成后,每2秒自动切换一 张图片。 (2)切换图片时,其对应按钮的样式同步变 换一背景颜色变为红色。 (3)当鼠标悬停在某个按钮上时,显示该按钮对应的图片且轮播停止。使用html和js编写代码 实现三张图的轮播效果
时间: 2024-02-11 13:08:25 浏览: 25
由于无法显示图9-59,我将根据您的描述进行实现。以下是代码:
HTML:
```html
<div id="slider">
<img src="image1.jpg" class="slider-image">
<img src="image2.jpg" class="slider-image">
<img src="image3.jpg" class="slider-image">
<div id="slider-buttons">
<button class="slider-button"></button>
<button class="slider-button"></button>
<button class="slider-button"></button>
</div>
</div>
```
CSS:
```css
#slider {
position: relative;
height: 400px;
width: 600px;
overflow: hidden;
}
.slider-image {
position: absolute;
top: 0;
left: 0;
height: 100%;
width: 100%;
opacity: 0;
transition: opacity 1s;
}
.slider-image.active {
opacity: 1;
}
#slider-buttons {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.slider-button {
height: 10px;
width: 10px;
border-radius: 50%;
background-color: #ccc;
margin: 0 10px;
outline: none;
border: none;
cursor: pointer;
}
.slider-button.active {
background-color: red;
}
```
JavaScript:
```javascript
const sliderImages = document.querySelectorAll(".slider-image");
const sliderButtons = document.querySelectorAll(".slider-button");
let currentIndex = 0;
let intervalId;
// 切换图片
function changeImage(index) {
sliderImages[currentIndex].classList.remove("active");
sliderButtons[currentIndex].classList.remove("active");
currentIndex = index;
sliderImages[currentIndex].classList.add("active");
sliderButtons[currentIndex].classList.add("active");
}
// 自动轮播
function startCarousel() {
intervalId = setInterval(() => {
let nextIndex = currentIndex + 1;
if (nextIndex >= sliderImages.length) {
nextIndex = 0;
}
changeImage(nextIndex);
}, 2000);
}
// 停止轮播
function stopCarousel() {
clearInterval(intervalId);
}
// 初始化
function init() {
startCarousel();
sliderButtons.forEach((button, index) => {
button.addEventListener("click", () => {
stopCarousel();
changeImage(index);
});
button.addEventListener("mouseover", () => {
stopCarousel();
changeImage(index);
});
button.addEventListener("mouseout", () => {
startCarousel();
});
});
}
init();
```
解释说明:
1. HTML中`#slider`为轮播图容器,其中包含三张图片和三个按钮,按钮通过`#slider-buttons`容器包裹。
2. CSS中设置轮播图容器为相对定位,图片为绝对定位,同时初始时所有图片的透明度为0。按钮设置为绝对定位,水平居中。
3. JavaScript中首先获取所有图片和按钮的DOM元素,同时定义一个变量`currentIndex`记录当前显示的图片下标,定义一个变量`intervalId`记录轮播定时器的ID。
4. `changeImage`函数用于切换图片,根据传入的下标修改当前显示的图片,同时修改对应的按钮样式。
5. `startCarousel`函数用于开启自动轮播,通过`setInterval`每2秒调用一次`changeImage`函数切换图片。
6. `stopCarousel`函数用于停止自动轮播,通过`clearInterval`清除定时器。
7. `init`函数用于初始化轮播图,为每个按钮绑定点击、鼠标悬停和鼠标移出事件,通过调用`changeImage`函数切换图片,同时开启自动轮播。
相关推荐
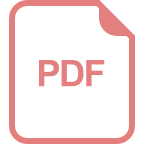
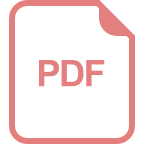














