帮我写一个rasa后端训练程序,可以连接到前端的Rasa Web Chat项目
时间: 2024-03-16 22:47:11 浏览: 14
好的,下面是一个简单的 Rasa 后端训练程序的示例,您可以参考并根据自己的需求进行修改和扩展。这个示例程序使用了 Rasa 2.x 版本,包括了自然语言理解 (NLU) 和对话管理 (DM) 的功能,并且可以连接到前端的 Rasa Web Chat 项目,提供智能问答服务。
首先,您需要安装 Rasa 和相关的依赖库。您可以使用以下命令来安装:
```
pip install rasa[spacy]
python -m spacy download en_core_web_md
```
然后,您需要编写培训数据,定义意图和实体,并编写自定义操作。这里我们使用一个简单的培训数据,包括一些常见的问答对。您可以将其保存为 `data/nlu.md` 文件。
```
## intent:greet
- hello
- hi
- hey
- good morning
- good afternoon
- good evening
## intent:goodbye
- bye
- goodbye
- see you
- see you later
## intent:thanks
- thank you
- thanks
- thank you very much
- thanks a lot
## intent:ask_name
- what is your name
- who are you
- what should I call you
- can you introduce yourself
## intent:ask_age
- how old are you
- what is your age
- when were you born
## intent:ask_location
- where are you from
- where do you live
- what is your location
## intent:ask_weather
- what is the weather like today
- is it going to rain today
- how hot is it today
## intent:default
- anything else
- what else can you do
- I have a question
```
接下来,您需要定义意图和实体,以及编写自定义操作。这里我们使用了一些简单的意图和实体,并提供了一些自定义操作,用于处理用户的输入和生成相应的回答。您可以将其保存为 `domain.yml` 文件。
```
intents:
- greet
- goodbye
- thanks
- ask_name
- ask_age
- ask_location
- ask_weather
- default
entities:
- location
- date
responses:
utter_greet:
- text: "Hello, how can I help you?"
utter_goodbye:
- text: "Goodbye, have a nice day!"
utter_thanks:
- text: "You're welcome, happy to help!"
utter_ask_name:
- text: "My name is ChatBot, what's yours?"
utter_ask_age:
- text: "I'm a chatbot, so I don't have an age. How can I assist you?"
utter_ask_location:
- text: "I'm a virtual assistant, so I don't have a physical location. What can I do for you?"
utter_ask_weather:
- text: "I'm sorry, I'm not able to provide real-time weather information at the moment."
utter_default:
- text: "I'm not sure I understand. Can you please rephrase your question?"
```
最后,您需要编写 Rasa 后端程序的代码,启动 Rasa 服务器,并提供 API 接口,用于处理用户的输入和生成相应的回答。这里我们使用了一个简单的 Flask 应用程序,您可以将其保存为 `app.py` 文件。
```
from flask import Flask, request, jsonify
from rasa.core.agent import Agent
app = Flask(__name__)
agent = Agent.load("models/20210205-005303.tar.gz")
@app.route("/webhooks/rasa/webhook", methods=["POST"])
def webhook():
data = request.json
message = data["message"]
sender_id = data["sender"]
response = agent.handle_text(message, sender_id=sender_id)
text = response[0]["text"]
return jsonify({"text": text})
if __name__ == "__main__":
app.run(debug=True)
```
在上面的代码中,我们首先创建了一个 Flask 应用程序,并加载了之前训练好的 Rasa 模型。然后,我们定义了一个 API 接口,并使用 `handle_text()` 方法处理用户的输入,生成相应的回答。最后,我们启动了 Flask 应用程序,并开启了调试模式。
在启动后端程序之前,您还需要先通过 `rasa train` 命令来训练 Rasa 模型,并生成相应的模型文件。在训练完成后,您可以将模型文件保存到 `models` 目录下,并使用 `tar.gz` 格式进行压缩。然后,您可以使用以下命令来启动后端程序:
```
python app.py
```
启动后,在您的前端 Rasa Web Chat 项目中,您可以使用以下代码来连接到后端程序,并与聊天机器人进行交互:
```
const widget = window.WebChat.create({
socketUrl: "http://localhost:5000/webhooks/rasa/webhook",
title: "ChatBot",
initPayload: "/greet",
inputTextFieldHint: "请输入您的问题...",
connectingText: "正在连接到聊天机器人...",
showFullScreenButton: true,
hideWhenNotConnected: false,
embedded: true,
params: {
storage: "session"
}
});
window.WebChat.open("chat-bot", widget, () => {
console.log("聊天窗口已打开!");
});
```
在上面的代码中,我们使用了 `socketUrl` 参数指定了 Rasa 后端程序的地址和端口号,并使用了一些其他的配置选项,例如聊天窗口的标题、提示文本、样式等等。然后,我们调用了 `window.WebChat.open()` 方法,将聊天窗口嵌入到网页中,并指定了一个 DOM 元素作为容器。
相关推荐
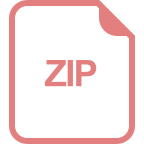
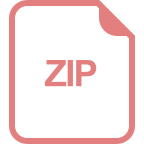
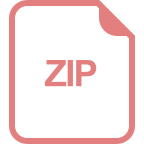














