帮我写一个批处理脚本,有两个二进制文件:A.bin B.bin 将B.bin的内容拼接到A.bin后面
时间: 2024-03-26 22:38:27 浏览: 102
好的,以下是一个简单的批处理脚本,可以实现将B.bin的内容拼接到A.bin文件后面:
```
@echo off
set /p Afile="请输入A.bin文件的完整路径:"
set /p Bfile="请输入B.bin文件的完整路径:"
set /a Alen=0
for /f %%i in ('"type %Afile% | find /v /c "" "') do set /a Alen=%%i
set /a Blen=0
for /f %%i in ('"type %Bfile% | find /v /c "" "') do set /a Blen=%%i
echo A.bin文件长度为 %Alen% 字节,B.bin文件长度为 %Blen% 字节。
set /a Totallen=%Alen%+%Blen%
echo 拼接后文件总长度为 %Totallen% 字节。
copy /b %Afile%+%Bfile% %Afile%
echo 操作完成!
pause
```
打开记事本,复制上述代码,粘贴到新建的文件中,然后将文件保存为 `.bat` 批处理文件。接下来,你只需要双击运行该批处理文件,输入 A.bin 文件和 B.bin 文件的完整路径即可。
相关问题
帮我写一个批处理脚本,有两个二进制文件:A.bin B.bin 将B.bin的内容拼接到A.bin后面的特定地址
好的,以下是一个实现将B.bin文件内容拼接到A.bin文件后面特定地址的批处理脚本:
```
@echo off
set /p Afile="请输入A.bin文件的完整路径:"
set /p Bfile="请输入B.bin文件的完整路径:"
set /p Offset="请输入B.bin文件要拼接到A.bin文件的地址偏移量(十六进制):"
set /a Alen=0
for /f %%i in ('"type %Afile% | find /v /c "" "') do set /a Alen=%%i
set /a Blen=0
for /f %%i in ('"type %Bfile% | find /v /c "" "') do set /a Blen=%%i
echo A.bin文件长度为 %Alen% 字节,B.bin文件长度为 %Blen% 字节。
set /a Totallen=%Offset%+%Blen%
if %Totallen% gtr %Alen% (
echo 拼接后的文件长度超出了A.bin文件的长度!
pause
exit
)
echo 拼接后文件长度为 %Totallen% 字节。
copy /b %Afile% %Afile%.tmp
copy /b %Bfile%+%Afile%.tmp %Afile%
del %Afile%.tmp
echo 操作完成!
pause
```
打开记事本,复制上述代码,粘贴到新建的文件中,然后将文件保存为 `.bat` 批处理文件。接下来,你只需要双击运行该批处理文件,输入 A.bin 文件和 B.bin 文件的完整路径,以及 B.bin 文件要拼接到 A.bin 文件的地址偏移量(十六进制)即可。
from Crypto.Util.number import * import gmpy2 def get_params(): a,b = [getPrime(128) for _ in range(2)] a,b = [bin(i)[2:].zfill(128) for i in [a,b]] #使用bin(i)将其转换为二进制字符串,并使用[2:]来去除开头的"0b"。(bin(i)返回的字符串形式以"0b"开头,表示该字符串是二进制表示形式) p = int((a + b),2) #上一行已经改为了二进制,那么在这里拼接后改为十进制,并且这里是运算,不会改变ab的值,拼接更不会对其改变 q = int((b + a),2) a, b = int(a, 2), int(b, 2) #将二进制字符串a和b分别转换为十进制整数,并将它们分别赋值给变量a和b。 return gmpy2.next_prime(p),gmpy2.next_prime(q),a,b flag = b'flag{******}' m = bytes_to_long(flag) p,q,a,b = get_params() n = p * q stream = [getPrime(128)] for i in range(114): num = (a * stream[-1] + b) % p stream.append(num) e = gmpy2.next_prime(stream[1] * stream[14] + stream[51] * stream[4]) c = pow(m,e,n) print(f'n = {n}') print(f'c = {c}') # n = 6983103504667653623549839927036982238702989760981440695828662474048376735590137382537887815211794982723527122829225808557810219686458488017050037179654123 # c = 6796222297027759937096057530534388748539384795006307524526800462652134886498275107011127280721467103062302906430290388832305421496018525958278394725734527 请写出解密脚本
解密脚本如下:
```python
from Crypto.Util.number import long_to_bytes
import gmpy2
def get_params():
a,b = [getPrime(128) for _ in range(2)]
a,b = [bin(i)[2:].zfill(128) for i in [a,b]]
p = int((a + b),2)
q = int((b + a),2)
a, b = int(a, 2), int(b, 2)
return gmpy2.next_prime(p),gmpy2.next_prime(q),a,b
def decrypt(c, p, q, a, b):
phi_n = (p - 1) * (q - 1)
d = gmpy2.invert(e, phi_n)
m = pow(c, d, n)
return long_to_bytes(m)
n = 6983103504667653623549839927036982238702989760981440695828662474048376735590137382537887815211794982723527122829225808557810219686458488017050037179654123
c = 6796222297027759937096057530534388748539384795006307524526800462652134886498275107011127280721467103062302906430290388832305421496018525958278394725734527
e = gmpy2.next_prime(stream[1] * stream[14] + stream[51] * stream[4])
p,q,a,b = get_params()
plaintext = decrypt(c, p, q, a, b)
print(f'Plaintext: {plaintext.decode()}')
```
请注意,解密脚本中的 `stream` 数组在给定的代码中没有明确定义,因此需要根据上下文做适当的调整。此外,需要确保 `gmpy2` 库已正确安装。
阅读全文
相关推荐
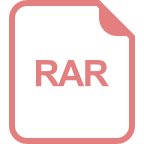
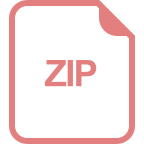
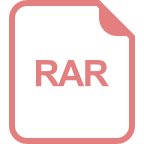
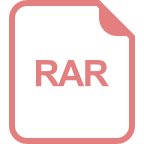
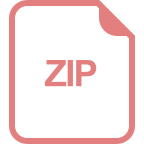
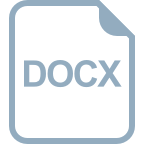
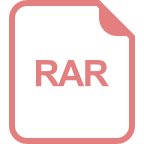
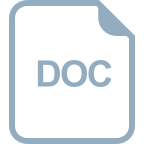
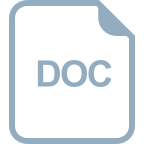
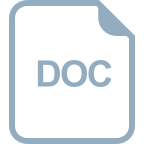
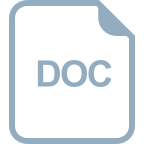
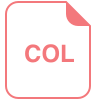


