java word转pdf
时间: 2023-09-13 11:05:19 浏览: 77
### 回答1:
在 Java 中转换 Word 文件为 PDF 文件,有几种方法可以考虑:
1. 使用 Apache POI 库:Apache POI 是一个开源的 Java 库,可以用于读写 Microsoft Office 文件(包括 Word、Excel、PowerPoint 等)。使用 POI,可以先读取 Word 文件内容,然后使用 iText 库将内容写入 PDF 文件中。
2. 使用 Aspose.Words 库:Aspose.Words 是一个专业的文档处理库,提供了丰富的 API,可以用于读写各种文档格式(包括 Word、Excel、PDF 等)。使用 Aspose.Words 可以直接将 Word 文件转换为 PDF 文件。
3. 使用 Microsoft Office Interop 库:如果机器上安装了 Microsoft Office,还可以使用 Microsoft Office Interop 库来转换 Word 文件为 PDF。这种方法的缺点是需要在机器上安装 Microsoft Office,并且代码依赖于特定版本的 Office。
综上,使用 Apache POI 和 Aspose.Words 库是比较方便和灵活的选择,可以在不安装 Microsoft Office 的情况下实现 Word 转 PDF 功能。
### 回答2:
在Java中实现Word转PDF的功能,可以通过借助一些第三方库来实现。以下是一种可能的方法,使用Apache POI和iText库实现:
1. 首先,需要引入Apache Poi和iText库的依赖。
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
```
2. 创建一个Java类,例如`WordToPdfConverter`,编写一个静态方法`convert`来实现转换功能。
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.converter.pdf.PdfConverter;
import org.apache.poi.xwpf.converter.pdf.PdfOptions;
public class WordToPdfConverter {
public static void convert(String wordFilePath, String pdfFilePath) throws Exception {
// 加载Word文档
FileInputStream in = new FileInputStream(wordFilePath);
XWPFDocument document = new XWPFDocument(in);
// 创建PDF选项
PdfOptions options = PdfOptions.create();
// 将Word文档转换为PDF
FileOutputStream out = new FileOutputStream(pdfFilePath);
PdfConverter.getInstance().convert(document, out, options);
// 关闭流
document.close();
in.close();
out.close();
}
}
```
3. 调用`WordToPdfConverter`的`convert`方法来实现转换。
```java
public class Main {
public static void main(String[] args) {
String wordFilePath = "input.docx"; // Word文件路径
String pdfFilePath = "output.pdf"; // 要保存的PDF文件路径
try {
WordToPdfConverter.convert(wordFilePath, pdfFilePath);
System.out.println("成功将Word文档转换为PDF文件!");
} catch (Exception e) {
System.out.println("转换失败:" + e.getMessage());
}
}
}
```
以上就是一个简单的使用Java实现Word转PDF的方法。需要注意的是,该方法依赖于Apache POI和iText库,因此需要将对应的依赖项添加到项目中才能正常运行。
### 回答3:
Java可以使用Apache POI库来实现Word文档转换为PDF文档的功能。Apache POI是一个开源的Java库,用于处理Microsoft Office格式的文档。
首先,我们需要添加Apache POI库的依赖到我们的Java项目中。可以通过在项目的Maven配置文件中添加以下依赖项来实现:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
然后,我们可以使用POI库中的XWPFDocument类来读取Word文档。我们可以使用以下代码来读取Word文档:
```
FileInputStream fis = new FileInputStream("input.docx");
XWPFDocument document = new XWPFDocument(fis);
```
接下来,我们可以使用Apache PDFBox库来将XWPFDocument对象转换为PDF文件。我们需要添加以下依赖项到我们的项目中:
```
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.24</version>
</dependency>
```
然后,我们可以使用PDFBox库中的PDFMergerUtility类来实现Word转PDF的功能。我们可以使用以下代码来将XWPFDocument对象转换为PDF文件:
```
FileOutputStream fos = new FileOutputStream("output.pdf");
PDFMergerUtility merger = new PDFMergerUtility();
merger.setDestinationStream(fos);
PDFFloatConverter converter = new PDFFloatConverter();
for (XWPFParagraph paragraph : document.getParagraphs()) {
PDFFontConverter fontConverter = new PDFFontConverter();
PDFStyledTextConverter textConverter = new PDFStyledTextConverter();
textConverter.setFontConverter(fontConverter);
List<XWPFRun> runs = paragraph.getRuns();
for (XWPFRun run : runs) {
String text = run.getText(0);
if (text != null) {
float fontSize = run.getFontSize();
String fontName = run.getFontFamily();
PDFFont font = fontConverter.convert(fontName, fontSize);
float spacing = run.getCharacterSpacing();
List<PdfTextChunk> chunks = textConverter.convert(text, font, spacing);
for (PdfTextChunk chunk : chunks) {
merger.addSource(chunk.getText());
}
}
}
}
merger.mergeDocuments();
fos.close();
```
最后,我们通过运行上述代码即可将Word文档转换为PDF文件,并保存在指定的路径上。
相关推荐
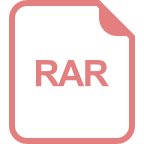





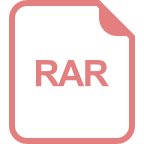
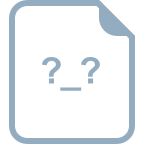
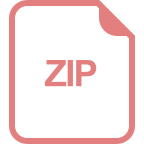
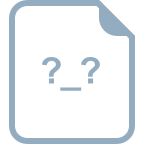
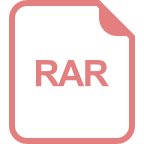