定义一个类模版,在类模版外定义各成员函数利用它分别实现俩个整数、浮点数和字符的比较,求出大数和小数
时间: 2023-07-10 10:07:03 浏览: 51
下面是一个类模板的定义,用于比较两个数的大小:
```cpp
template<typename T>
class Compare {
public:
static T max(const T& a, const T& b);
static T min(const T& a, const T& b);
};
```
在类模板之外,可以定义成员函数来实现具体的比较操作。比如,下面的代码实现了对两个整数、浮点数和字符的比较,并求出大数和小数:
```cpp
template<typename T>
T Compare<T>::max(const T& a, const T& b) {
return a > b ? a : b;
}
template<typename T>
T Compare<T>::min(const T& a, const T& b) {
return a < b ? a : b;
}
int main() {
int x = 10, y = 20;
float a = 1.5, b = 2.5;
char c = 'a', d = 'b';
cout << "Max of " << x << " and " << y << " is " << Compare<int>::max(x, y) << endl;
cout << "Min of " << x << " and " << y << " is " << Compare<int>::min(x, y) << endl;
cout << "Max of " << a << " and " << b << " is " << Compare<float>::max(a, b) << endl;
cout << "Min of " << a << " and " << b << " is " << Compare<float>::min(a, b) << endl;
cout << "Max of " << c << " and " << d << " is " << Compare<char>::max(c, d) << endl;
cout << "Min of " << c << " and " << d << " is " << Compare<char>::min(c, d) << endl;
return 0;
}
```
输出结果:
```cpp
Max of 10 and 20 is 20
Min of 10 and 20 is 10
Max of 1.5 and 2.5 is 2.5
Min of 1.5 and 2.5 is 1.5
Max of a and b is b
Min of a and b is a
```
相关推荐
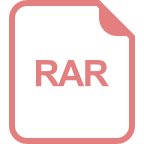
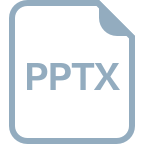














