QTimer larmda
时间: 2024-01-22 18:02:42 浏览: 69
QTimer是Qt中的一个计时器类,可以用来定时触发某些操作。lambda表达式是C++11中的一个特性,可以用来定义匿名函数。
在使用QTimer时,可以使用lambda表达式来定义计时器触发时执行的操作。例如:
```cpp
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, [=](){
qDebug() << "Timer triggered!";
});
timer->start(1000); // 每隔1秒触发一次计时器
```
上述代码中,我们创建了一个QTimer对象,并连接了它的timeout信号到一个lambda表达式中。这个lambda表达式会在计时器触发时执行,输出一条调试信息。
在lambda表达式中,我们使用了捕获列表[=],表示捕获当前作用域下的所有变量。这样就可以在lambda表达式中使用当前作用域下的变量,比如QObject子类中的this指针。
注意,在使用lambda表达式时,需要确保编译器支持C++11标准。
相关问题
Pyqt QTimer
PyQt QTimer 是 PyQt 库中的一个类,用于创建定时器对象,可以用来触发定时事件。它可以在指定的时间间隔内发出 timeout 信号。
要使用 QTimer,首先需要导入 PyQt 模块:
```python
from PyQt5.QtCore import QTimer
```
然后可以创建一个 QTimer 对象,并设置定时器的间隔(以毫秒为单位):
```python
timer = QTimer()
timer.setInterval(1000) # 设置定时器间隔为 1 秒
```
接下来,你可以连接 timeout 信号到一个槽函数,当定时器超时时,该槽函数将被调用:
```python
def on_timeout():
print("Timeout!")
timer.timeout.connect(on_timeout)
```
最后,你可以启动定时器:
```python
timer.start()
```
当定时器运行时,每当经过指定的时间间隔后,timeout 信号将被发送,从而触发绑定的槽函数。
qtimer setsingleshot
QTimer is a class in Qt framework which is used to create and manage timers. The setSingleShot() function is a method of QTimer class which is used to set the timer to be a single-shot timer.
A single-shot timer is a timer which fires only once after the specified interval and then stops. It is useful when we want to perform a certain task after a specific delay or after a certain event occurs.
The syntax of the setSingleShot() function is as follows:
```cpp
void QTimer::setSingleShot(bool singleShot)
```
where singleShot is a boolean value which specifies whether the timer should be a single-shot timer or not. If it is set to true, then the timer will be a single-shot timer, otherwise it will be a repetitive timer.
For example, the following code snippet creates a single-shot timer which will fire after 5 seconds:
```cpp
QTimer *timer = new QTimer(this);
timer->setSingleShot(true);
timer->start(5000); // 5000 milliseconds = 5 seconds
connect(timer, &QTimer::timeout, [=]() {
qDebug() << "Timer expired!";
});
```
In this example, we create a QTimer object and set it to be a single-shot timer using the setSingleShot(true) function. Then we start the timer with a delay of 5 seconds using the start(5000) function. Finally, we connect the timeout() signal of the timer to a lambda function which will print a message to the console when the timer expires.
阅读全文
相关推荐
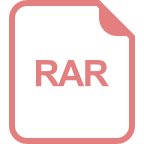
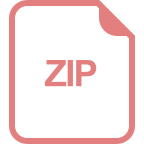












