String hash = Md5Algorithm.getInstance().md5Digest(sign_str.getBytes(StandardCharsets.UTF_8)); log.info(String.format("签名处理中,签名源内容:%s,对应MD5值:%s", sign_str, hash)); PKCS8EncodedKeySpec priPKCS8 = new PKCS8EncodedKeySpec(Base64.getBytesBASE64(prikeyvalue)); KeyFactory keyf = KeyFactory.getInstance("RSA"); PrivateKey myprikey = keyf.generatePrivate(priPKCS8); // 用私钥对信息生成数字签名 java.security.Signature signet = java.security.Signature.getInstance("MD5withRSA"); signet.initSign(myprikey); signet.update(sign_str.getBytes(StandardCharsets.UTF_8)); byte[] signed = signet.sign(); // 对信息的数字签名 return new String(org.apache.commons.codec.binary.Base64.encodeBase64(signed)); 上面的代码用python怎么实现?
时间: 2023-03-05 10:36:03 浏览: 249
以下是使用Python进行等价操作的代码示例:
```python
import hashlib
import base64
from Crypto.PublicKey import RSA
from Crypto.Signature import pkcs1_15
# 计算字符串的 MD5 哈希值
sign_str = "your_sign_str"
hash = hashlib.md5(sign_str.encode('utf-8')).hexdigest()
print("签名处理中,签名源内容:{},对应MD5值:{}".format(sign_str, hash))
# 解析 RSA 私钥
prikeyvalue = "your_private_key_in_base64_format"
prikeyvalue_bytes = base64.b64decode(prikeyvalue)
private_key = RSA.import_key(prikeyvalue_bytes)
# 使用私钥对消息进行签名
signer = pkcs1_15.new(private_key)
signature = signer.sign(hashlib.md5(sign_str.encode('utf-8')))
signature_b64 = base64.b64encode(signature).decode('utf-8')
return signature_b64
```
需要注意的是,在 Python 中实现 RSA 签名时,需要使用 PyCryptodome 或 PyCrypto 等第三方库,因为 Python 自带的 `rsa` 模块不支持 PKCS#1 v1.5 签名。上面的代码示例使用了 PyCryptodome。另外,如果你需要使用的是 PEM 格式的 RSA 私钥,需要使用 PyCryptodome 中的 `Crypto.PublicKey.RSA.import_key` 方法进行解析。
阅读全文
相关推荐
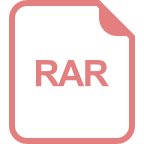
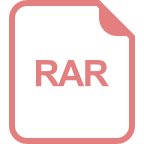
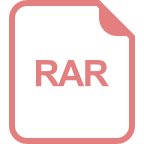

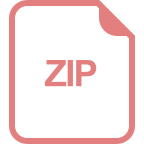
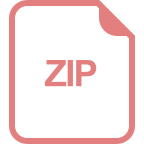
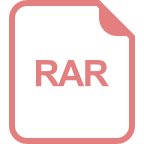
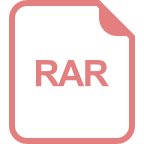
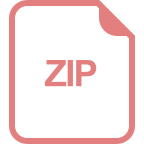
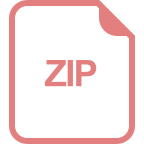
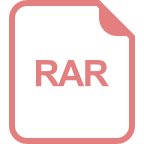
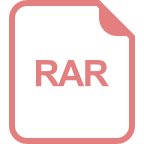
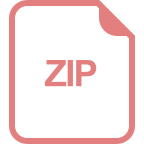
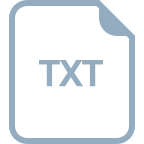
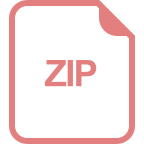
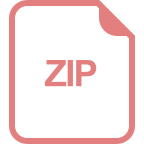
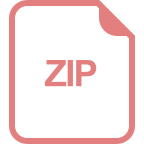

