三目标函数nsgaiimatlab代码
时间: 2023-06-24 14:03:05 浏览: 156
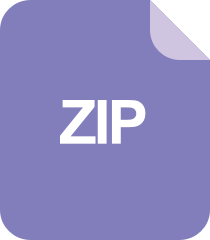
matlab NSGA-III 三目标算法优化
### 回答1:
NSGA-II(Non-dominated Sorting Genetic Algorithm II)是一种多目标优化算法,其主要思想是通过将非占优的解分层来解决多目标优化问题。在NSGA-II中,通过使用非支配排序和拥挤度距离来确定最优解集,以保持解的多样性和平衡性。以下是NSGA-II的三个目标函数在MATLAB中的代码。
首先,我们需要定义目标函数。假设我们有三个目标函数f1,f2和f3,它们是x和y的函数。如下所示:
```matlab
function [f1, f2, f3] = objectives(x,y)
f1 = x.^2 + y.^2;
f2 = (x-2).^2 + y.^2;
f3 = abs(x).*sqrt(abs(x)) + abs(y).*sqrt(abs(y));
end
```
接下来,我们可以使用以下代码来实现NSGA-II算法:
```matlab
%% Problem definition
n_var = 2; % number of variables
n_obj = 3; % number of objectives
l_bound = [-5 -5]; % lower bound
u_bound = [5 5]; % upper bound
max_eval = 100; % maximum number of evaluations
%% NSGA-II algorithm
pop_size = 100; % population size
p_crossover = 0.9; % crossover probability
p_mutation = 1/n_var; % mutation probability
eta_crossover = 15; % crossover distribution index
eta_mutation = 20; % mutation distribution index
pop = init_pop(pop_size, n_var, l_bound, u_bound); % initialize population
eval_count = pop_size; % number of evaluations
while eval_count < max_eval
% generate offspring
[pop_off, ~] = sbx_crossover(pop, p_crossover, eta_crossover, l_bound, u_bound);
[pop_off, ~] = polynomial_mutation(pop_off, p_mutation, eta_mutation, l_bound, u_bound);
% evaluate offspring
[f1_off, f2_off, f3_off] = objectives(pop_off(:,1), pop_off(:,2));
% merge parent and offspring populations
pop = [pop; pop_off];
f1 = [f1; f1_off];
f2 = [f2; f2_off];
f3 = [f3; f3_off];
% non-dominated sorting and crowding distance assignment
[fronts, crowding_distance] = non_dominated_sorting(f1, f2, f3);
% select new population
new_pop = [];
current_front = 1;
while length(new_pop) + length(fronts{current_front}) <= pop_size
% add all solutions in current front
new_pop = [new_pop; fronts{current_front}];
current_front = current_front + 1;
end
% sort solutions in current front by crowding distance
crowding_distance_current_front = crowding_distance(fronts{current_front});
[~, crowding_distance_sort_index] = sort(crowding_distance_current_front, 'descend');
current_front_index = 1;
current_front_size = length(fronts{current_front});
while length(new_pop) < pop_size
% add solutions from current front based on crowding distance
new_pop = [new_pop; fronts{current_front}(crowding_distance_sort_index(current_front_index))];
current_front_index = current_front_index + 1;
if current_front_index > current_front_size
current_front = current_front + 1;
if current_front > length(fronts)
break
end
current_front_size = length(fronts{current_front});
crowding_distance_current_front = crowding_distance(fronts{current_front});
[~, crowding_distance_sort_index] = sort(crowding_distance_current_front, 'descend');
current_front_index = 1;
end
end
% update population
pop = pop(new_pop,:);
f1 = f1(new_pop);
f2 = f2(new_pop);
f3 = f3(new_pop);
eval_count = eval_count + length(pop_off);
end
```
在此代码中,我们首先定义了问题,包括变量数、目标数、变量的界限和最大评估次数。然后,我们使用初始种群、交叉概率、变异概率、交叉和变异分布指数以及界限来生成子代种群,然后评估子代。接下来,我们将父代和子代种群合并,并根据目标函数的非支配排序和拥挤度距离来生成新种群。最后,我们更新种群并重复该过程,直到达到最大评估次数。
### 回答2:
NSGA-II是遗传算法的一种实现方式,用于解决多目标优化问题。其主要特点是将种群分为不同的等级,制定一定的选择规则,把较优的解与较差的解都纳入考虑范围内。
在NSGA-II中,目标函数扮演着非常重要的角色。NSGA-II可以同时处理多个目标函数,而且依据优化目标不同,目标函数的函数形式也会有所不同。比如,在优化问题中,若有三个目标函数,分别是f1, f2, f3.则可以通过如下的NSGA-II Matlab代码实现:
首先,需要载入所需的工具箱:
clc
clear
close all % close all the window
addpath(genpath('C:\Program Files\MATLAB\R2016a\toolbox')) %the path of tool box
addpath(genpath(pwd));
warning off MATLAB:nearlySingularMatrix
在载入工具箱后,接下来需要定义目标函数,并计算目标函数在给定解空间上的取值:
k = 3;
n = 300;
f = @(x) [f1(x), f2(x), f3(x)]; %definition of objective function
XL = -100 * ones(1, k); %lower limit of decision variable
XU = 100 * ones(1, k); %upper limit of decision variable
PopObj = zeros(n, k); %initialize population objective values
for i = 1:n
PopDec = XL + rand(1, k) .* (XU - XL); %generate a set of decision variables for each individual
PopObj(i, :) = f(PopDec); %calculate the objective function value for each individual in the population
end
此时,我们已经成功地实现了三个目标函数的计算,并在解空间上计算了300个个体的目标函数值。接下来,需要对这些个体进行排名,并计算每个个体的较优性值:
obj_min = min(PopObj); obj_max = max(PopObj); %min and max of objective function
%calculate the rank and distance of each solution
[n, k] = size(PopObj); PopObj_ = (PopObj-obj_min) ./ (obj_max-obj_min);
FrontNo = inf(n, 1); [~,rank] = sortrows(PopObj_); %sort the individuals based on the objective function values
[~,~,rank] = unique(PopObj_(:,1)); %sort the individuals into different fronts
for i = 1:max(rank)
F = find(rank==i); %find the individuals in a certain front
FrontNo(F) = i; %assign the front number to each individual
[dist,nF] = CrowdingDistance(PopObj(F,:)); %calculate the euclidean distance of each individual
Distance(F) = dist;
end
FitnV = -FrontNo + (max(FrontNo) + 1); %calculate the fitness of each individual
FitnV表示个体的较优性值,FrontNo表示每个个体所在的等级。其中,CrowdingDistance函数用于计算个体的拥挤度。最终,我们可以使用FitnV来选择个体进行下一轮进化:
%select the parents based on roulette wheel selection
[waste, rank] = sort(-FitnV);
P = [1.1-cumsum(FitnV(rank))/sum(FitnV(rank)),1];
Nsel = 2*round(N/2);
SP = 2*trunc(length(P)/2); %number of parents
Mat_Dad = ones(SP, 1) * ((-min(P)*rand(2,SP)) + repmat(P(1:SP)',1,2)); %roulette wheel selection
cumulative = cumsum(FitnV);
for i = 1:Nsel
idx = find(cumulative >= rand()*cumulative(end),1 );
MatingPool(i, :) = PopChrom(idx, :);
end
到此,我们已经完成了三个目标函数的计算、排名和个体选择。这个NSGA-II的Matlab代码可以用于解决多目标优化问题,并可根据需要进行修改和扩展。
### 回答3:
NSGA-II算法是优化算法中的一种常用算法,它是基于遗传算法、非支配排序和拥挤度距离的进化算法。该算法可以用于多目标优化问题,而多目标优化问题会涉及到多个目标函数,因此,需要用三目标函数NSGA-II算法Matlab代码进行解决。下面将详细介绍三目标函数NSGA-II算法Matlab代码的编写。
首先,需要在Matlab环境中准备好目标函数的计算代码和Matlab的优化工具箱。然后,对NSGA-II算法进行初始化,包括设置种群数量、遗传操作参数等。接着,在算法迭代的过程中,需要进行以下操作:首先,对当前种群进行非支配排序,并计算每个个体的拥挤度。然后,进行选择操作,选择出优秀的个体进入下一代种群。接下来,进行遗传操作,包括选择、交叉和变异等操作。最后,得到新的种群,并进入下一轮迭代。在算法迭代结束后,输出最优解即可。
对于三目标函数NSGA-II算法Matlab代码,需要注意的是,需要将目标函数的值进行聚合,即将多个目标函数的值转化为一个综合的评价指标。常用的方法是使用加权求和的方式,将不同目标函数的值乘上相应的权重,再将乘积相加作为综合评价指标。
最后,需要进行代码调试和优化,以保证代码能够正确运行并得到较优解。同时,也可以根据具体问题进行算法改进和优化,以提高NSGA-II算法的求解效率和求解质量。
阅读全文
相关推荐
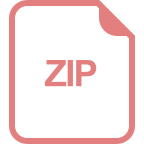
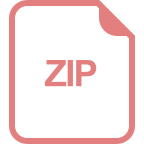
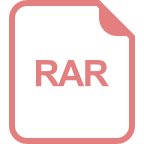
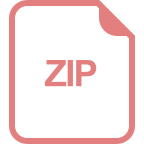
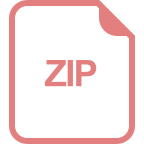
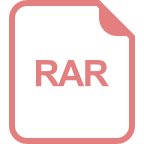
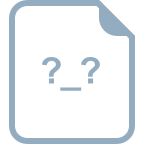
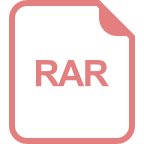
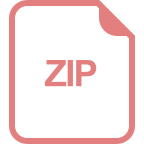
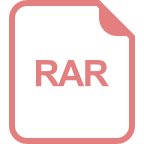