编写一个自动发送微信信息的python小程序
时间: 2023-09-20 10:06:04 浏览: 118
### 回答1:
你好,我是 C 知道,关于你的问题,我可以回答。编写一个自动发送微信信息的 Python 小程序,可以使用 wxpy 库来实现。首先需要安装 wxpy 库,然后使用登录微信账号的方式来获取登录凭证,接着可以使用 wxpy 库提供的 send() 方法来发送微信信息。具体实现细节可以参考 wxpy 官方文档。希望能对你有所帮助。
### 回答2:
编写一个自动发送微信信息的Python小程序可以分为以下几个步骤:
1. 导入所需的库和模块:使用`itchat`库来实现微信的登录和发送消息功能。可以使用`pip`命令安装该库。
2. 登录微信账号:通过`itchat.auto_login()`函数实现微信账号的登录。该函数会生成一个登录二维码,用户使用手机微信扫描二维码登录。
3. 搜索好友或群组:使用`itchat.search_friends()`或`itchat.search_chatrooms()`函数来搜索需要发送消息的好友或群组。可以根据好友或群组的名称、备注等进行搜索。
4. 发送消息:使用`itchat.send()`函数来发送消息。该函数接受参数包括要发送的消息内容、接收方(好友或群组)的UserName等信息。
5. 编写主程序:在主程序中,可以实现以下功能:
- 调用`auto_login()`函数登录微信账号。
- 利用`search_friends()`或`search_chatrooms()`函数搜索需要发送消息的对象。
- 使用`send()`函数发送消息。
以下是一个示例程序:
```python
import itchat
# 登录微信账号
itchat.auto_login()
# 搜索好友或群组
friend = itchat.search_friends(name='好友名称')[0] # 根据好友的备注名称进行搜索
group = itchat.search_chatrooms(name='群组名称')[0] # 根据群组的名称进行搜索
# 发送消息
itchat.send('你好,好友', toUserName=friend['UserName']) # 发送给好友
itchat.send('大家好,这是自动发送的消息', toUserName=group['UserName']) # 发送给群组
```
这样,当运行该程序时,就能自动登录并发送消息给指定好友或群组。注意,该程序需要保持运行状态,才能自动发送消息。如果希望定时发送消息,可以结合Python的定时任务模块(如`schedule`)来实现。
### 回答3:
编写一个自动发送微信信息的Python小程序可以使用`itchat2`库实现。以下是一个简单的代码示例:
```python
import itchat
# 登录微信账号
itchat.auto_login()
# 定义自动发送消息的函数
def send_message(friend_name, message):
# 获取好友列表
friends = itchat.get_friends()
# 遍历好友列表,找到目标好友
for friend in friends:
if friend['RemarkName'] == friend_name or friend['NickName'] == friend_name:
# 获取目标好友的微信号
friend_username = friend['UserName']
# 发送消息
itchat.send(message, toUserName=friend_username)
print('消息已发送给%s:%s' % (friend_name, message))
return
# 如果未找到目标好友,则打印错误消息
print('未找到好友:%s' % friend_name)
# 调用自动发送消息的函数,传入好友姓名和消息内容
send_message('好友姓名', '你好,这是一条自动发送的消息。')
# 等待函数运行完成后,保持程序运行,以便接收消息
itchat.run()
```
在使用该程序之前,需要通过手机微信扫描登录。然后,可以调用`send_message`函数发送消息给指定好友。程序会自动搜索好友列表中的备注名或昵称匹配的好友,并发送消息给该好友。
需要注意的是,该程序中的`itchat`库是基于itchat的第二个版本itchat2,因为微信更新后第一个版本已经无法正常登录。可以通过`pip install itchat2`命令安装该库。
此外,发送消息后,程序使用`itchat.run()`来保持程序的运行,并等待接收消息。可以在程序的其他地方添加逻辑以便处理接收到的消息。
相关推荐
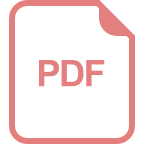
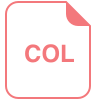
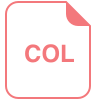
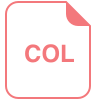
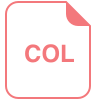
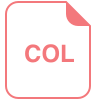









