pytorch怎么更改device的GPU数量
时间: 2023-08-31 21:38:10 浏览: 47
### 回答1:
要更改PyTorch中使用的GPU数量,可以使用以下代码:
```python
import torch
# 将模型放在第一个GPU上
device = torch.device("cuda:0")
# 更改GPU数量
torch.cuda.set_device(device)
# 确认更改
print(torch.cuda.current_device())
```
使用`torch.cuda.set_device()`函数,可以将GPU的索引号传递给它,从而更改PyTorch使用的GPU数量。在上面的示例中,我们将PyTorch设置为使用第一个GPU,并打印当前设备的索引以确认更改。
### 回答2:
在PyTorch中,可以通过以下步骤更改设备的GPU数量:
1. 导入必要的库:
```
import torch
```
2. 检查当前设备是否支持GPU:
```
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
```
3. 如果当前设备支持GPU,则可以使用以下命令更改GPU数量:
```
torch.cuda.set_device(device_id)
```
这里`device_id`是你想要使用的GPU的索引号,比如如果你有两个GPU,索引号分别为0和1,则可以使用`torch.cuda.set_device(1)`来将当前设备切换到第二个GPU。
4. 确认更改后的设备:
```
print(torch.cuda.current_device())
```
此命令将输出当前设备的索引号。
需要注意的是,一旦切换了设备,后续在PyTorch中创建的张量和模型都将在指定的设备上进行计算。如果需要在多个GPU上进行并行计算,还可以使用`torch.nn.DataParallel`来自动将模型拆分到各个GPU上进行计算。
### 回答3:
要更改PyTorch中的GPU数量,可以按照以下步骤进行操作:
1. 首先,检查当前可用的GPU设备数量。使用`torch.cuda.device_count()`函数可以获取当前系统上可用的GPU设备数量。
2. 然后,使用`torch.cuda.set_device(device_id)`函数将当前设备设置为要使用的GPU设备。`device_id`参数为GPU设备的索引号,从0开始编号。例如,要将设备设置为第一个GPU设备,可以使用`torch.cuda.set_device(0)`。
3. 如果需要使用多个GPU设备,可以使用`torch.cuda.device(device_id)`函数切换到其他设备。同样,`device_id`参数为GPU设备的索引号。
下面是一个例子,演示了如何修改PyTorch中的GPU设备数量:
```python
import torch
# 获取当前可用的GPU设备数量
num_gpus = torch.cuda.device_count()
print("可用的GPU设备数量:", num_gpus)
# 将当前设备设置为第一个GPU设备
torch.cuda.set_device(0)
print("当前设备:", torch.cuda.current_device())
# 切换到第二个GPU设备
torch.cuda.device(1)
print("当前设备:", torch.cuda.current_device())
```
需要注意的是,修改GPU设备数量只是更改了PyTorch在训练和推理过程中使用的设备数量,而不是实际改变了系统上的GPU硬件数量。
相关推荐
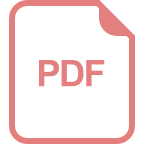
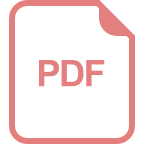
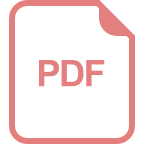
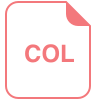
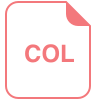
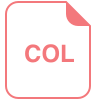
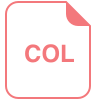
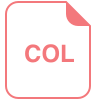









